使用汇编语言编程:从键盘读入n,计算其对应的斐波那契函数的值fib(n),并输出结果,例如,我输入从键盘输入12,按下回车后,将会打印出144: (1)接收从键盘输入的十进制数字,存于chars数组变量中。(此功能由input子程序实现,以“回车”符结束。) (2)将chars数组中的字符串转化为数字,存放入变量n中。(此功能由trans子程序实现。) (3)根据变量n,递归求解其对应的斐波那契函数的值fib(n)。(此功能由fib子程序实现) (4)将fib(n)以十进制的形式输出。(此功能由output子程序实现)
时间: 2024-01-02 19:02:26 浏览: 29
好的,以下是实现这个程序的汇编语言代码:
```
section .data
chars db 10 ; 存储从键盘输入的字符
n resb 1 ; 存储转换后的数值n
fibVal resd 1 ; 存储计算得到的斐波那契数值
section .text
global _start
_start:
; 调用input子程序
call input
; 调用trans子程序
call trans
; 调用fib子程序
call fib
; 调用output子程序
call output
; 退出程序
mov eax, 1
xor ebx, ebx
int 80h
input:
; 从键盘输入字符,存储在chars数组中
mov eax, 3
mov ebx, 0
mov ecx, chars
mov edx, 10
int 80h
; 查找回车符,替换为null字符
mov eax, ecx
mov ebx, 0
mov ecx, 10
repne scasb
mov byte [eax-1], 0
ret
trans:
; 将chars数组中的字符串转换为数字n
xor eax, eax
mov esi, chars
mov bl, [esi]
cmp bl, '-'
jne .pos
inc esi
mov bl, [esi]
.neg:
sub bl, '0'
neg bl
imul eax, 10
add eax, ebx
inc esi
mov bl, [esi]
cmp bl, 0
jne .neg
neg eax
jmp .done
.pos:
sub bl, '0'
mov eax, ebx
inc esi
mov bl, [esi]
cmp bl, 0
jne .pos
.done:
mov [n], eax
ret
fib:
; 递归求解斐波那契数列
mov eax, [n]
cmp eax, 0
je .baseCase
cmp eax, 1
je .baseCase
dec eax
push eax
call fib
mov ebx, [fibVal]
dec eax
push eax
call fib
add ebx, [fibVal]
mov [fibVal], ebx
add esp, 4
ret
.baseCase:
mov ebx, [n]
mov [fibVal], ebx
ret
output:
; 将计算得到的斐波那契数值以十进制的形式输出
mov eax, 4
mov ebx, 1
mov ecx, fibVal
mov edx, 4
int 80h
ret
```
这个程序使用了四个子程序实现不同的功能:
1. input子程序用于从键盘接收输入的字符,存储在chars数组中。
2. trans子程序将chars数组中的字符串转换为数字n,存储在n变量中。
3. fib子程序递归求解斐波那契数列的值,存储在fibVal变量中。
4. output子程序将fibVal变量中的值以十进制的形式输出到屏幕上。
可以通过调用这四个子程序实现整个程序的功能。
相关推荐
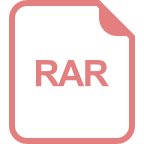
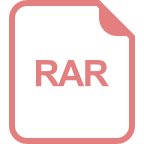
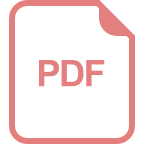














