在Spring Boot框架下的web评论系统,实体有评论、子评论、用户和回复,实现浏览、发布、更新和删除功能。
时间: 2023-06-18 08:08:39 浏览: 56
好的,首先你需要定义四个实体类,分别为Comment、SubComment、User和Reply。其中Comment和SubComment是一对多的关系(即一个Comment下可以有多个SubComment),SubComment和Reply也是一对多的关系(即一个SubComment下可以有多个Reply),User则是一对多的关系(即一个User可以对应多个Comment和SubComment)。
下面是针对这四个实体类的建表语句:
```
CREATE TABLE `user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(255) NOT NULL,
`password` varchar(255) NOT NULL,
PRIMARY KEY (`id`)
);
CREATE TABLE `comment` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`content` varchar(255) NOT NULL,
`user_id` int(11) NOT NULL,
PRIMARY KEY (`id`),
FOREIGN KEY (`user_id`) REFERENCES `user`(`id`)
);
CREATE TABLE `sub_comment` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`content` varchar(255) NOT NULL,
`comment_id` int(11) NOT NULL,
`user_id` int(11) NOT NULL,
PRIMARY KEY (`id`),
FOREIGN KEY (`comment_id`) REFERENCES `comment`(`id`),
FOREIGN KEY (`user_id`) REFERENCES `user`(`id`)
);
CREATE TABLE `reply` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`content` varchar(255) NOT NULL,
`sub_comment_id` int(11) NOT NULL,
`user_id` int(11) NOT NULL,
PRIMARY KEY (`id`),
FOREIGN KEY (`sub_comment_id`) REFERENCES `sub_comment`(`id`),
FOREIGN KEY (`user_id`) REFERENCES `user`(`id`)
);
```
接下来是Spring Boot框架下的web评论系统的实现:
1. 在pom.xml文件中添加依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
```
2. 在application.properties文件中添加数据库连接信息:
```
spring.datasource.url=jdbc:mysql://localhost:3306/comment_system
spring.datasource.username=root
spring.datasource.password=123456
spring.jpa.hibernate.ddl-auto=update
```
3. 编写实体类:
User.java
```java
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
@OneToMany(mappedBy = "user", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private List<Comment> comments;
@OneToMany(mappedBy = "user", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private List<SubComment> subComments;
@OneToMany(mappedBy = "user", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private List<Reply> replies;
// 省略getter和setter
}
```
Comment.java
```java
@Entity
@Table(name = "comment")
public class Comment {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String content;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
@OneToMany(mappedBy = "comment", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private List<SubComment> subComments;
// 省略getter和setter
}
```
SubComment.java
```java
@Entity
@Table(name = "sub_comment")
public class SubComment {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String content;
@ManyToOne
@JoinColumn(name = "comment_id")
private Comment comment;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
@OneToMany(mappedBy = "subComment", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private List<Reply> replies;
// 省略getter和setter
}
```
Reply.java
```java
@Entity
@Table(name = "reply")
public class Reply {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String content;
@ManyToOne
@JoinColumn(name = "sub_comment_id")
private SubComment subComment;
@ManyToOne
@JoinColumn(name = "user_id")
private User user;
// 省略getter和setter
}
```
4. 编写Repository:
UserRepository.java
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
```
CommentRepository.java
```java
@Repository
public interface CommentRepository extends JpaRepository<Comment, Long> {
}
```
SubCommentRepository.java
```java
@Repository
public interface SubCommentRepository extends JpaRepository<SubComment, Long> {
}
```
ReplyRepository.java
```java
@Repository
public interface ReplyRepository extends JpaRepository<Reply, Long> {
}
```
5. 编写Service:
UserService.java
```java
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public User save(User user) {
return userRepository.save(user);
}
public User findById(Long id) {
return userRepository.findById(id).orElse(null);
}
public List<User> findAll() {
return userRepository.findAll();
}
public void delete(Long id) {
userRepository.deleteById(id);
}
}
```
CommentService.java
```java
@Service
public class CommentService {
@Autowired
private CommentRepository commentRepository;
public Comment save(Comment comment) {
return commentRepository.save(comment);
}
public Comment findById(Long id) {
return commentRepository.findById(id).orElse(null);
}
public List<Comment> findAll() {
return commentRepository.findAll();
}
public void delete(Long id) {
commentRepository.deleteById(id);
}
}
```
SubCommentService.java
```java
@Service
public class SubCommentService {
@Autowired
private SubCommentRepository subCommentRepository;
public SubComment save(SubComment subComment) {
return subCommentRepository.save(subComment);
}
public SubComment findById(Long id) {
return subCommentRepository.findById(id).orElse(null);
}
public List<SubComment> findAll() {
return subCommentRepository.findAll();
}
public void delete(Long id) {
subCommentRepository.deleteById(id);
}
}
```
ReplyService.java
```java
@Service
public class ReplyService {
@Autowired
private ReplyRepository replyRepository;
public Reply save(Reply reply) {
return replyRepository.save(reply);
}
public Reply findById(Long id) {
return replyRepository.findById(id).orElse(null);
}
public List<Reply> findAll() {
return replyRepository.findAll();
}
public void delete(Long id) {
replyRepository.deleteById(id);
}
}
```
6. 编写Controller:
UserController.java
```java
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@PostMapping("/save")
public User save(@RequestBody User user) {
return userService.save(user);
}
@GetMapping("/find/{id}")
public User findById(@PathVariable("id") Long id) {
return userService.findById(id);
}
@GetMapping("/find-all")
public List<User> findAll() {
return userService.findAll();
}
@DeleteMapping("/delete/{id}")
public void delete(@PathVariable("id") Long id) {
userService.delete(id);
}
}
```
CommentController.java
```java
@RestController
@RequestMapping("/comment")
public class CommentController {
@Autowired
private CommentService commentService;
@PostMapping("/save")
public Comment save(@RequestBody Comment comment) {
return commentService.save(comment);
}
@GetMapping("/find/{id}")
public Comment findById(@PathVariable("id") Long id) {
return commentService.findById(id);
}
@GetMapping("/find-all")
public List<Comment> findAll() {
return commentService.findAll();
}
@DeleteMapping("/delete/{id}")
public void delete(@PathVariable("id") Long id) {
commentService.delete(id);
}
}
```
SubCommentController.java
```java
@RestController
@RequestMapping("/sub-comment")
public class SubCommentController {
@Autowired
private SubCommentService subCommentService;
@PostMapping("/save")
public SubComment save(@RequestBody SubComment subComment) {
return subCommentService.save(subComment);
}
@GetMapping("/find/{id}")
public SubComment findById(@PathVariable("id") Long id) {
return subCommentService.findById(id);
}
@GetMapping("/find-all")
public List<SubComment> findAll() {
return subCommentService.findAll();
}
@DeleteMapping("/delete/{id}")
public void delete(@PathVariable("id") Long id) {
subCommentService.delete(id);
}
}
```
ReplyController.java
```java
@RestController
@RequestMapping("/reply")
public class ReplyController {
@Autowired
private ReplyService replyService;
@PostMapping("/save")
public Reply save(@RequestBody Reply reply) {
return replyService.save(reply);
}
@GetMapping("/find/{id}")
public Reply findById(@PathVariable("id") Long id) {
return replyService.findById(id);
}
@GetMapping("/find-all")
public List<Reply> findAll() {
return replyService.findAll();
}
@DeleteMapping("/delete/{id}")
public void delete(@PathVariable("id") Long id) {
replyService.delete(id);
}
}
```
7. 编写前端页面:
在src/main/resources/static目录下添加index.html文件,内容如下:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Web评论系统</title>
</head>
<body>
<h1>Web评论系统</h1>
<h2>用户管理</h2>
<form id="user-form">
<label for="username">用户名:</label>
<input type="text" id="username" name="username">
<br>
<label for="password">密码:</label>
<input type="password" id="password" name="password">
<br>
<button type="button" onclick="saveUser()">保存</button>
</form>
<table id="user-table">
<thead>
<tr>
<th>id</th>
<th>用户名</th>
<th>密码</th>
<th>操作</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
<h2>评论管理</h2>
<form id="comment-form">
<label for="content">评论内容:</label>
<input type="text" id="content" name="content">
<br>
<label for="user-id">用户ID:</label>
<input type="text" id="user-id" name="user-id">
<br>
<button type="button" onclick="saveComment()">保存</button>
</form>
<table id="comment-table">
<thead>
<tr>
<th>id</th>
<th>评论内容</th>
<th>用户ID</th>
<th>操作</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
<h2>子评论管理</h2>
<form id="sub-comment-form">
<label for="content">子评论内容:</label>
<input type="text" id="content" name="content">
<br>
<label for="comment-id">评论ID:</label>
<input type="text" id="comment-id" name="comment-id">
<br>
<label for="user-id">用户ID:</label>
<input type="text" id="user-id" name="user-id">
<br>
<button type="button" onclick="saveSubComment()">保存</button>
</form>
<table id="sub-comment-table">
<thead>
<tr>
<th>id</th>
<th>子评论内容</th>
<th>评论ID</th>
<th>用户ID</th>
<th>操作</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
<h2>回复管理</h2>
<form id="reply-form">
<label for="content">回复内容:</label>
<input type="text" id="content" name="content">
<br>
<label for="sub-comment-id">子评论ID:</label>
<input type="text" id="sub-comment-id" name="sub-comment-id">
<br>
<label for="user-id">用户ID:</label>
<input type="text" id="user-id" name="user-id">
<br>
<button type="button" onclick="saveReply()">保存</button>
</form>
<table id="reply-table">
<thead>
<tr>
<th>id</th>
<th>回复内容</th>
<th>子评论ID</th>
<th>用户ID</th>
<th>操作</th>
</tr>
</thead>
<tbody>
</tbody>
</table>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
function saveUser() {
$.ajax({
url: "/user/save",
type: "POST",
dataType: "json",
contentType: "application/json;charset=utf-8",
data: JSON.stringify({
username: $("#username").val(),
password: $("#password").val()
}),
success: function (result) {
alert("保存成功!");
$("#user-form")[0].reset();
loadUserTable();
}
});
}
function loadUserTable() {
$.ajax({
url: "/user/find-all",
type: "GET",
dataType: "json",
success: function (result) {
var tbody = $("#user-table tbody");
tbody.empty();
$.each(result, function (index, item) {
var tr = $("<tr></tr>");
tr.append($("<td></td>").text(item.id));
tr.append($("<td></td>").text(item.username));
tr.append($("<td></td>").text(item.password));
var td = $("<td></td>");
td.append($("<button type='button'>删除</button>").click(function () {
deleteUser(item.id);
}));
tr.append(td);
tbody.append(tr);
});
}
});
}
function deleteUser(userId) {
$.ajax({
url: "/user/delete/" + userId,
type: "DELETE",
success: function () {
alert("删除成功!");
loadUserTable();
}
});
}
function saveComment() {
$.ajax({
url: "/comment/save",
type: "POST",
dataType: "json",
contentType: "application/json;charset=utf-8",
data: JSON.stringify({
content: $("#content").val(),
user: {id: $("#user-id").val()}
}),
success: function (result) {
alert("保存成功!");
$("#comment-form")[0].reset();
loadCommentTable();
}
});
}
function loadCommentTable() {
$.ajax({
url: "/comment/find-all",
type: "GET",
dataType: "json",
success: function (result) {
var tbody = $("#comment-table tbody");
tbody.empty();
$.each(result, function (index, item) {
var tr = $("<tr></tr>");
tr.append($("<td></td>").text(item.id));
tr.append($("<td></td>").text(item.content));
tr.append($("<td></td>").text(item.user.id));
var td = $("<td></td>");
td.append($("<button type='button'>删除</button>").click(function () {
deleteComment(item.id);
}));
tr.append(td);
tbody.append(tr);
});
}
});
}
function deleteComment(commentId) {
$.ajax({
url: "/comment/delete/" + commentId,
type: "DELETE",
success: function () {
alert("删除成功!");
loadCommentTable();
}
});
}
function saveSubComment() {
$.ajax({
url: "/sub-comment/save",
type: "POST",
dataType: "json",
contentType: "application/json;charset=utf-8",
data: JSON.stringify({
content: $("#content").val(),
comment: {id: $("#comment-id").val()},
user: {id: $("#user-id").val()}
}),
success: function (result) {
alert("保存成功!");
$("#sub-comment-form")[0].reset();
loadSubCommentTable();
}
});
}
function loadSubCommentTable() {
$.ajax({
url: "/sub-comment/find-all",
type: "GET",
dataType: "json",
success: function (result) {
var tbody = $("#sub-comment-table tbody");
tbody.empty();
$.each(result, function (index, item) {
var tr = $("<tr></tr>");
tr.append($("<td></td>").text(item.id));
tr.append($("<td></td>").text(item.content));
tr.append($("<td></td>").text(item.comment.id));
tr.append($("<td></td>").text(item.user.id));
var td = $("<td></td>");
td.append($("<button type='button'>删除</button>").click(function () {
deleteSubComment(item.id);
}));
tr.append(td);
tbody.append(tr);
});
}
});
}
function deleteSubComment(subCommentId) {
$.
相关推荐
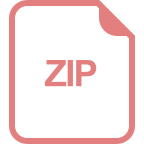
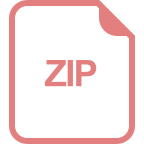
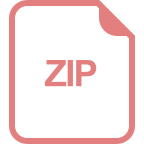














