python zhang-suen骨架算法实现
时间: 2023-08-07 12:21:15 浏览: 140
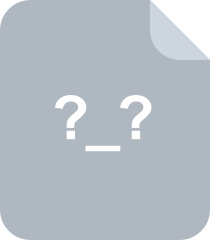
python骨架提取算法
以下是Python实现的Zhang-Suen骨架算法的示例代码:
``` python
import numpy as np
def ZhangSuen(image):
"""
Implementation of Zhang-Suen thinning algorithm for binary images.
"""
# Define the 8-neighborhood of a pixel
neighbors = ((-1,-1),(-1,0),(-1,1),(0,-1),(0,1),(1,-1),(1,0),(1,1))
# Initialize the image to be thinned
thinned_image = image.copy()
# Initialize flag to indicate if a pixel was removed
pixel_removed = True
while pixel_removed:
pixel_removed = False
# Step 1: Mark pixels to be removed
marked_pixels = []
for x in range(1, image.shape[0]-1):
for y in range(1, image.shape[1]-1):
if image[x,y] == 0:
continue
neighbors_count = sum([image[x+i,y+j] == 255 for i,j in neighbors])
if neighbors_count < 2 or neighbors_count > 6:
continue
transitions_count = sum([1 for i in range(8) if image[x+neighbors[i][0],y+neighbors[i][1]] == 0 and image[x+neighbors[i+1 if i<7 else 0][0],y+neighbors[i+1 if i<7 else 0][1]] == 255])
if transitions_count != 1:
continue
marked_pixels.append((x,y))
# Step 2: Remove marked pixels
for x,y in marked_pixels:
thinned_image[x,y] = 0
pixel_removed = True
# Step 3: Mark pixels to be removed
marked_pixels = []
for x in range(1, image.shape[0]-1):
for y in range(1, image.shape[1]-1):
if image[x,y] == 0:
continue
neighbors_count = sum([image[x+i,y+j] == 255 for i,j in neighbors])
if neighbors_count < 2 or neighbors_count > 6:
continue
transitions_count = sum([1 for i in range(8) if image[x+neighbors[i][0],y+neighbors[i][1]] == 0 and image[x+neighbors[i+1 if i<7 else 0][0],y+neighbors[i+1 if i<7 else 0][1]] == 255])
if transitions_count != 1:
continue
marked_pixels.append((x,y))
# Step 4: Remove marked pixels
for x,y in marked_pixels:
thinned_image[x,y] = 0
pixel_removed = True
return thinned_image
```
解释一下上面的代码:
首先,我们定义了一个8邻域的元组neighbors,表示一个像素的8个邻居像素;
然后,我们初始化一个待细化的二值图像thinned_image,复制输入的二值图像;
接着,我们开始迭代细化过程,直到没有像素可以被删除为止;
在每一轮迭代中,我们执行两个步骤:标记像素和删除像素。标记像素的过程先扫描一遍图像,找到满足一定条件的像素,并将它们加入到一个列表marked_pixels中。删除像素的过程再扫描一遍图像,将marked_pixels中的像素删除。这两个步骤分别执行两次,以保证算法的正确性;
标记像素的条件包括:它周围有2到6个前景像素;它周围的前景像素中只有一个像素的背景像素是它的邻居;它不是端点像素(即它的周围不是一个完整的线段);
删除像素的条件与标记像素的条件相同。
最后,我们返回细化后的二值图像。
阅读全文
相关推荐
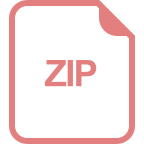
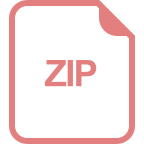

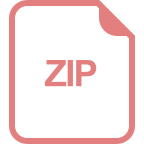
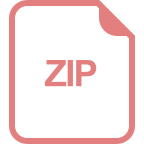
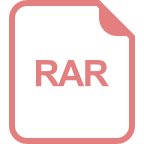
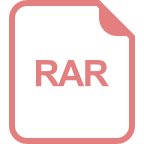
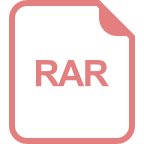
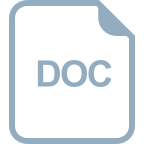
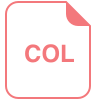



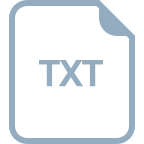
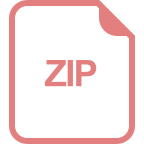
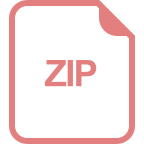
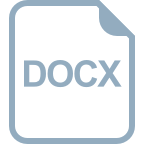
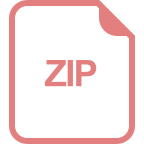