一个list里面有5个size为[1, 3, 64, 64]的tensor构成,怎么把这个list转为一个[5, 3, 64, 64]的tensor
时间: 2024-05-06 13:18:13 浏览: 12
你可以使用 PyTorch 中的 `torch.stack()` 函数来实现。`torch.stack()` 可以按照指定的维度将一个 list 中的 tensor 进行合并。具体操作如下:
```python
import torch
# 创建一个包含 5 个 tensor 的 list
tensor_list = [torch.randn(1, 3, 64, 64) for _ in range(5)]
# 使用 torch.stack() 将这 5 个 tensor 合并为一个 4 维 tensor
tensor_4d = torch.stack(tensor_list, dim=0)
# 查看合并后的 tensor 的 shape
print(tensor_4d.shape) # 输出: torch.Size([5, 3, 64, 64])
```
在上面的代码中,`torch.randn(1, 3, 64, 64)` 用于创建一个随机的 4 维 tensor。`range(5)` 创建一个包含 5 个数字的 range 对象,用于循环 5 次,创建 5 个 tensor。`torch.stack(tensor_list, dim=0)` 将这 5 个 tensor 按照 dim=0 维度合并为一个 4 维 tensor。最后输出合并后的 tensor 的 shape 为 [5, 3, 64, 64]。
相关问题
将一个list的ndarray转为tensor
可以使用PyTorch中的torch.tensor()函数将一个list的ndarray转为tensor。具体代码如下:
import torch
import numpy as np
# 创建一个list的ndarray
arr = np.array([1, 2, 3, 4, 5])
# 将ndarray转为tensor
tensor = torch.tensor(arr)
print(tensor)
输出结果为:
tensor([1, 2, 3, 4, 5])
paddlepaddle如何把一个列表转为图像tensor
首先,将列表转化为numpy数组,然后使用Pillow或OpenCV等图像处理库将numpy数组转化为图像。接着,使用PaddlePaddle的`fluid.dygraph.to_variable()`方法将图像转化为图像tensor。以下是示例代码:
```python
import numpy as np
from PIL import Image
import paddle.fluid as fluid
# 假设有一个包含图像路径的列表
image_list = ["path/to/image1.jpg", "path/to/image2.jpg", "path/to/image3.jpg"]
# 将图像列表转化为numpy数组
image_array = np.array([np.array(Image.open(img_path)) for img_path in image_list])
# 将numpy数组转化为图像tensor
image_tensor = fluid.dygraph.to_variable(image_array)
```
请注意,在将图像转化为numpy数组时,需要根据图像的颜色通道数进行相应的处理。例如,如果图像是RGB格式,则numpy数组的形状应为`(height, width, 3)`。
相关推荐
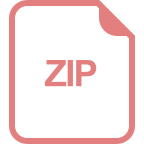












