springboot如何异步查询接口
时间: 2024-12-28 19:04:50 浏览: 5
在Spring Boot中,可以通过多种方式实现异步查询接口。以下是一种常见的方法,使用`@Async`注解和`CompletableFuture`类来实现异步查询:
1. **启用异步支持**:在Spring Boot应用的主类上添加`@EnableAsync`注解,以启用异步方法支持。
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableAsync;
@SpringBootApplication
@EnableAsync
public class AsyncApplication {
public static void main(String[] args) {
SpringApplication.run(AsyncApplication.class, args);
}
}
```
2. **创建异步服务**:在服务类中定义一个异步方法,并使用`@Async`注解标记该方法。确保该服务类被Spring管理(例如,使用`@Service`注解)。
```java
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
import java.util.concurrent.CompletableFuture;
@Service
public class AsyncService {
@Async
public CompletableFuture<String> asyncQuery() {
// 模拟异步操作,例如数据库查询或外部API调用
try {
Thread.sleep(5000); // 模拟耗时操作
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
return CompletableFuture.completedFuture("Async Result");
}
}
```
3. **创建控制器**:在控制器中调用异步服务的方法,并处理返回的`CompletableFuture`。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.concurrent.CompletableFuture;
@RestController
public class AsyncController {
@Autowired
private AsyncService asyncService;
@GetMapping("/async-query")
public CompletableFuture<String> asyncQuery() {
return asyncService.asyncQuery();
}
}
```
通过上述步骤,你就可以在Spring Boot应用中实现一个异步查询接口。访问`/async-query`接口时,服务器会立即返回`CompletableFuture`,而不会阻塞请求线程。
阅读全文
相关推荐
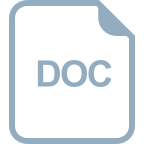
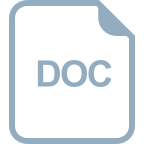
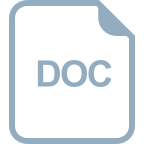

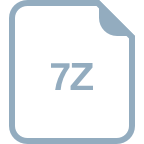

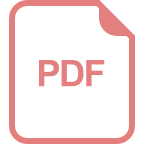
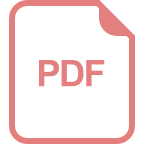
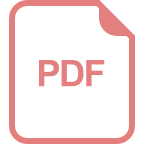









