已知PID和VID,如何使用WINUSB读取GUID
时间: 2024-05-01 13:21:14 浏览: 286
要使用WINUSB读取GUID,您需要执行以下步骤:
1. 打开设备
使用WinUsb_Initialize函数打开设备并获得设备句柄。您需要提供设备的PID和VID。
2. 获取GUID描述符
使用WinUsb_QueryDeviceInformation函数获取设备的GUID描述符。您需要指定GUID描述符的索引号。
3. 读取GUID
使用WinUsb_ReadPipe函数从设备中读取GUID。您需要指定读取的管道和缓冲区的大小。
以下是一个简单的代码示例,可帮助您开始使用WINUSB读取GUID:
```C++
#include <windows.h>
#include <winusb.h>
#include <setupapi.h>
#include <devguid.h>
#define GUID_INDEX 1
#define BUFFER_SIZE 16
int main()
{
// 打开设备
GUID guid;
HDEVINFO hdevinfo = NULL;
SP_DEVICE_INTERFACE_DATA interface_data;
SP_DEVINFO_DATA devinfo_data;
WINUSB_INTERFACE_HANDLE winusb_handle;
BOOL result;
// 获取设备信息
hdevinfo = SetupDiGetClassDevs(&GUID_DEVCLASS_USB, NULL, NULL, DIGCF_PRESENT | DIGCF_DEVICEINTERFACE);
if (hdevinfo == INVALID_HANDLE_VALUE) {
printf("Failed to get device info\n");
return 1;
}
// 枚举设备
interface_data.cbSize = sizeof(SP_DEVICE_INTERFACE_DATA);
result = SetupDiEnumDeviceInterfaces(hdevinfo, NULL, &GUID_DEVCLASS_USB, 0, &interface_data);
if (!result) {
printf("Failed to enum device interfaces\n");
return 1;
}
// 获取设备路径
DWORD required_size;
result = SetupDiGetDeviceInterfaceDetail(hdevinfo, &interface_data, NULL, 0, &required_size, NULL);
if (!result && GetLastError() != ERROR_INSUFFICIENT_BUFFER) {
printf("Failed to get device interface detail\n");
return 1;
}
PSP_DEVICE_INTERFACE_DETAIL_DATA interface_detail_data;
interface_detail_data = (PSP_DEVICE_INTERFACE_DETAIL_DATA)malloc(required_size);
interface_detail_data->cbSize = sizeof(SP_DEVICE_INTERFACE_DETAIL_DATA);
result = SetupDiGetDeviceInterfaceDetail(hdevinfo, &interface_data, interface_detail_data, required_size, NULL, NULL);
if (!result) {
printf("Failed to get device interface detail\n");
return 1;
}
// 打开 WinUSB 设备
HANDLE device_handle = CreateFile(interface_detail_data->DevicePath, GENERIC_READ | GENERIC_WRITE, FILE_SHARE_READ | FILE_SHARE_WRITE, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL | FILE_FLAG_OVERLAPPED, NULL);
if (device_handle == INVALID_HANDLE_VALUE) {
printf("Failed to open device\n");
return 1;
}
// 初始化 WinUSB
result = WinUsb_Initialize(device_handle, &winusb_handle);
if (!result) {
printf("Failed to initialize WinUSB\n");
CloseHandle(device_handle);
return 1;
}
// 获取 GUID 描述符
UCHAR guid_descriptor[BUFFER_SIZE];
ULONG guid_descriptor_length = BUFFER_SIZE;
result = WinUsb_QueryDeviceInformation(winusb_handle, DeviceUxdGuid, &guid_descriptor_length, guid_descriptor);
if (!result) {
printf("Failed to query GUID descriptor\n");
WinUsb_Free(winusb_handle);
CloseHandle(device_handle);
return 1;
}
// 读取 GUID
UCHAR guid_data[BUFFER_SIZE];
ULONG guid_length = BUFFER_SIZE;
result = WinUsb_ReadPipe(winusb_handle, 0x81, guid_data, guid_length, NULL, NULL);
if (!result) {
printf("Failed to read GUID\n");
WinUsb_Free(winusb_handle);
CloseHandle(device_handle);
return 1;
}
// 关闭设备
WinUsb_Free(winusb_handle);
CloseHandle(device_handle);
return 0;
}
```
阅读全文
相关推荐
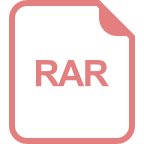
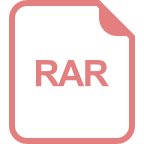
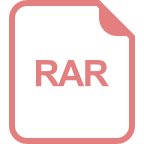
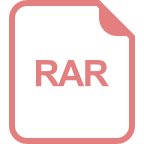
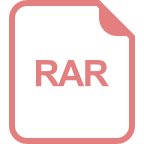
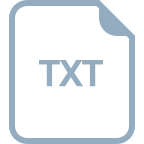
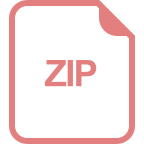
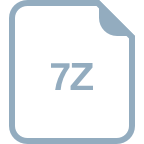
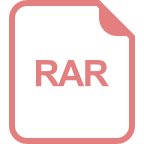
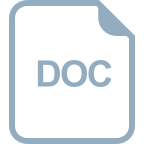
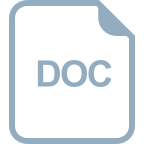




