写一个movies.csv的管理系统用c语言
时间: 2024-10-04 18:01:21 浏览: 27
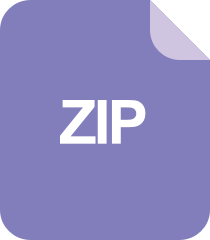
基于C语言实现电影推荐系统【100011950】
创建一个基于C语言的电影管理系统,通常会涉及到文件操作、数据结构以及简单的用户界面。这里是一个简化的例子,展示如何使用C语言来管理一个CSV格式的电影列表:
首先,我们假设CSV文件的结构如下:
- id (int)
- title (string)
- year (int)
- genre (string)
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义电影结构体
typedef struct {
int id;
char title[100];
int year;
char genre[50];
} Movie;
// 读取CSV文件并添加到电影数组
void read_movies(char *filename, Movie movies[], int *size) {
FILE *fp = fopen(filename, "r");
if (!fp) {
printf("Failed to open file.\n");
return;
}
*size = 0;
while (fgets(line, sizeof(line), fp)) {
char *endptr;
// 解析CSV行
int id = strtol(line, &endptr, 10);
if (*endptr == ',') {
strcpy(movies[*size].title, ++endptr);
endptr = strchr(endptr, ',');
movies[*size].year = strtol(endptr + 1, NULL, 10);
endptr = strchr(endptr + 1, ',');
strcpy(movies[*size].genre, ++endptr);
} else {
printf("Invalid CSV format.\n");
break;
}
movies[*size].id = id;
(*size)++;
}
fclose(fp);
}
// 显示电影列表
void display_movies(Movie movies[], int size) {
for (int i = 0; i < size; i++) {
printf("%d - %s (%d) [%s]\n", movies[i].id, movies[i].title, movies[i].year, movies[i].genre);
}
}
int main() {
const char *movie_file = "movies.csv";
int movie_count = 0;
// 初始化电影数组
Movie *movies = malloc(sizeof(Movie));
if (!movies) {
printf("Memory allocation failed.\n");
return 1;
}
// 读取并处理CSV文件
read_movies(movie_file, movies, &movie_count);
// 显示所有电影
display_movies(movies, movie_count);
// 添加删除等其他功能可以在此基础上扩展
free(movies);
return 0;
}
```
阅读全文
相关推荐
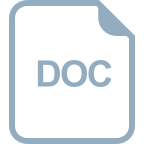
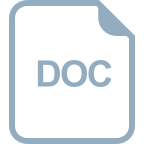
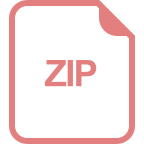
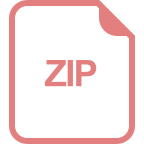








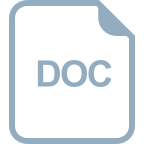
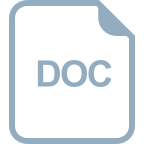
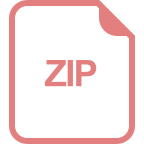
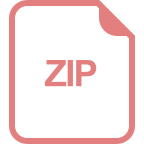