plt.plot() set_data
时间: 2023-09-15 13:21:01 浏览: 61
The `plt.plot()` function in Matplotlib is used to create a line plot or scatter plot. The `set_data()` method is used to set the data for the line or scatter plot.
For example, to create a line plot with x and y data and set the data using `set_data()`, you can use the following code:
```
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
fig, ax = plt.subplots()
line, = ax.plot(x, y)
# Set new data for line plot
x_new = [1, 2, 3, 4, 5]
y_new = [1, 3, 5, 7, 9]
line.set_data(x_new, y_new)
plt.show()
```
This will create a line plot with the original x and y data, and then set new data for the line plot using `set_data()` with `x_new` and `y_new`.
Similarly, you can use `set_data()` to set the data for a scatter plot. Here's an example:
```
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
fig, ax = plt.subplots()
scatter = ax.scatter(x, y)
# Set new data for scatter plot
x_new = [1, 2, 3, 4, 5]
y_new = [1, 3, 5, 7, 9]
scatter.set_offsets(list(zip(x_new, y_new)))
plt.show()
```
This will create a scatter plot with the original x and y data, and then set new data for the scatter plot using `set_offsets()` with `x_new` and `y_new`.
相关推荐
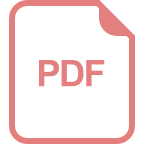














