import matplotlib.ticker as ticker # 设置图形大小 plt.figure(figsize=(10,6)) # 筛选出广东省的数据 guangdong_data = data[data['省'] == '广东省'] # 将日期列转换为年份列 guangdong_data['年份'] = pd.DatetimeIndex(guangdong_data['日期']).year # 计算每年的平均气温 yearly_temp = guangdong_data.groupby('年份')['平均气温'].mean() # 绘制线性图 plt.plot(yearly_temp.index, yearly_temp.values) plt.title('2000年到2019年广东省年平均气温') # 设置X轴刻度显示整数 plt.gca().xaxis.set_major_locator(ticker.MaxNLocator(integer=True)) plt.xlabel('年份') plt.ylabel('平均气温') plt.show()每行代码解释
时间: 2023-09-14 12:15:58 浏览: 47
这段代码是用来绘制广东省2000年到2019年的年平均气温的线性图,并且设置了图形的大小,X轴刻度显示整数。
- 第1行:导入matplotlib库中的ticker模块,用于设置图形的刻度。
- 第2行:设置图形的大小为10*6英寸(宽*高)。
- 第4行:从数据集data中筛选出省份为“广东省”的数据,保存到guangdong_data中。
- 第6行:利用pandas库中的DatetimeIndex函数将日期列转换为年份列,并添加到guangdong_data中。
- 第8行:对guangdong_data按照年份进行分组,并计算每年的平均气温,保存到yearly_temp中。
- 第11行:利用pyplot库中的plot函数绘制线性图,其中x轴为年份,y轴为平均气温。
- 第12行:设置图形的标题为“2000年到2019年广东省年平均气温”。
- 第14行:设置X轴刻度为整数,即只显示整数刻度。
- 第15行:设置x轴标签为“年份”。
- 第16行:设置y轴标签为“平均气温”。
- 第17行:利用pyplot库中的show函数显示图形。
相关问题
import matplotlib.ticker as ticker
Matplotlib.ticker是Matplotlib库中的一个模块,用于在图表中设置刻度定位器和格式化器。它可以帮助我们更好地控制坐标轴的显示效果。
常见的刻度定位器和格式化器包括:
1. FixedLocator:指定固定的刻度位置;
2. MultipleLocator:指定刻度间隔;
3. AutoLocator:自动选择刻度间隔;
4. NullLocator:不显示刻度;
5. FormatStrFormatter:用指定的格式化字符串格式化刻度值;
6. ScalarFormatter:用科学计数法格式化刻度值。
下面是一个简单示例,展示如何使用刻度定位器和格式化器:
```python
import matplotlib.pyplot as plt
import matplotlib.ticker as ticker
# 生成数据
x = [1, 2, 3, 4, 5]
y = [10, 20, 15, 25, 30]
# 绘制折线图
plt.plot(x, y)
# 设置x轴刻度定位器和格式化器
x_locator = ticker.MultipleLocator(1) # 设置间隔为1
x_formatter = ticker.FormatStrFormatter('%d') # 格式化为整数
plt.gca().xaxis.set_major_locator(x_locator)
plt.gca().xaxis.set_major_formatter(x_formatter)
# 设置y轴刻度定位器和格式化器
y_locator = ticker.MultipleLocator(5) # 设置间隔为5
y_formatter = ticker.ScalarFormatter() # 使用科学计数法
plt.gca().yaxis.set_major_locator(y_locator)
plt.gca().yaxis.set_major_formatter(y_formatter)
# 显示图形
plt.show()
```
运行代码后,会得到一个折线图,其中x轴的刻度间隔为1,格式为整数;y轴的刻度间隔为5,使用科学计数法显示。
import matplotlib.ticker as mtick
`matplotlib.ticker`是Matplotlib库中用于绘制坐标轴刻度线和标签的工具包。`mtick`模块提供了一些常用的刻度线格式化器和定位器。比如可以使用`PercentFormatter`来将小数转换为百分数形式,也可以使用`MultipleLocator`来设置坐标轴刻度的间隔。
以下是一个简单例子,演示如何使用`mtick`模块来设置y轴刻度为百分数形式:
``` python
import matplotlib.pyplot as plt
import matplotlib.ticker as mtick
import numpy as np
x = np.linspace(0, 1, 11)
y = np.random.rand(11)
fig, ax = plt.subplots()
ax.plot(x, y)
# 设置y轴刻度为百分数形式
fmt = '%.0f%%' # 将小数转换为百分数,保留0位小数
yticks = mtick.FormatStrFormatter(fmt)
ax.yaxis.set_major_formatter(yticks)
plt.show()
```
运行以上代码,将会得到一张图像,y轴刻度显示为百分数形式。
相关推荐
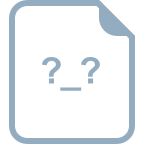
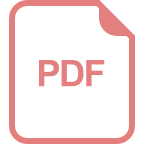
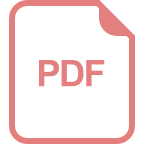












