pandas绘图下方折线图上方柱状图
时间: 2023-07-07 17:46:39 浏览: 55
您可以使用 matplotlib 库来实现 pandas 绘图下方折线图上方柱状图的效果。下面是一个简单的示例代码:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 创建示例数据
df = pd.DataFrame({
'日期': ['1月', '2月', '3月', '4月', '5月'],
'销售额': [100, 200, 300, 400, 500],
'利润率': [0.1, 0.15, 0.2, 0.25, 0.3]
})
# 绘制折线图
fig, ax1 = plt.subplots()
ax1.plot(df['日期'], df['销售额'], color='red', linewidth=2)
ax1.set_ylabel('销售额')
# 绘制柱状图
ax2 = ax1.twinx()
ax2.bar(df['日期'], df['利润率'], alpha=0.3)
ax2.set_ylabel('利润率')
# 设置图例
ax1.legend(['销售额'], loc='upper left')
ax2.legend(['利润率'], loc='upper right')
# 显示图形
plt.show()
```
运行上述代码,即可得到 pandas 绘图下方折线图上方柱状图的效果。您可以根据需要修改代码中的数据和参数来适应自己的需求。
相关问题
pandas画图折线图和柱状图,两个图结合的图
可以使用pandas中的plot函数绘制折线图和柱状图,并使用matplotlib库进行绘图。
例如,我们创建一个包含两列数据的DataFrame:
```python
import pandas as pd
import numpy as np
# 创建DataFrame
data = {'year': [2015, 2016, 2017, 2018, 2019],
'sales': [100, 150, 200, 180, 220],
'profit': [20, 30, 40, 35, 50]}
df = pd.DataFrame(data)
```
然后,我们可以使用plot函数绘制折线图和柱状图:
```python
import matplotlib.pyplot as plt
# 绘制折线图
df.set_index('year')['sales'].plot(kind='line', color='blue', label='Sales')
df.set_index('year')['profit'].plot(kind='line', color='red', label='Profit')
plt.ylabel('Amount')
plt.legend(loc='best')
plt.title('Sales and Profit')
# 绘制柱状图
df.set_index('year').plot(kind='bar', rot=0)
plt.ylabel('Amount')
plt.title('Sales and Profit')
# 结合两个图
fig, ax1 = plt.subplots()
ax1.plot(df['year'], df['sales'], color='blue')
ax1.set_ylabel('Sales', color='blue')
ax1.tick_params('y', colors='blue')
ax2 = ax1.twinx()
ax2.bar(df['year'], df['profit'], color='red', alpha=0.3)
ax2.set_ylabel('Profit', color='red')
ax2.tick_params('y', colors='red')
plt.title('Sales and Profit')
plt.show()
```
最后一个图中,我们使用了subplots函数创建一个包含两个y轴的子图,并在第一个y轴上绘制了折线图,在第二个y轴上绘制了柱状图。注意调整柱状图的透明度,以确保两个图形不会互相遮挡。
利用pandas绘图感悟
利用pandas进行数据可视化是一种非常方便和强大的方式。通过pandas的绘图功能,可以直接在数据分析的过程中进行数据可视化,帮助我们更好地理解和展示数据。
在使用pandas绘图时,我们可以使用DataFrame或Series对象的plot()方法来生成各种类型的图表,如折线图、柱状图、散点图等。同时,pandas还提供了一些参数和选项,可以对图表进行进一步的定制和美化。
通过利用pandas绘图,我深刻感悟到以下几点:
1. 数据可视化是数据分析的重要环节:通过绘制图表,我们可以更直观地观察数据的分布、趋势和关系,从而更好地理解数据背后的规律和趋势。
2. pandas的绘图功能简单易用:pandas提供了简洁而强大的绘图接口,使得我们能够快速生成各种类型的图表,并且可以通过参数和选项进行进一步的定制。
3. 数据可视化有助于沟通和展示结果:通过绘制图表,我们可以将复杂的数据分析结果以直观的方式展示给他人,提高沟通效果和结果的可解释性。
相关推荐
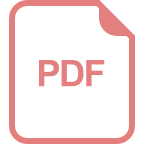
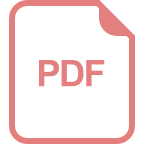












