opencv拟合圆
时间: 2023-07-04 13:26:26 浏览: 163
在OpenCV中,可以使用cv::fitEllipse()函数和cv::fitCircle()函数拟合椭圆和圆。
cv::fitEllipse()函数用于拟合椭圆,该函数的输入参数为点集,输出参数为椭圆的中心坐标、长轴半径、短轴半径和旋转角度。具体实现如下:
```cpp
#include <opencv2/opencv.hpp>
int main()
{
// 构造点集
std::vector<cv::Point2f> pts;
pts.push_back(cv::Point2f(50, 50));
pts.push_back(cv::Point2f(100, 100));
pts.push_back(cv::Point2f(150, 50));
pts.push_back(cv::Point2f(100, 0));
// 拟合椭圆
cv::RotatedRect ellipse = cv::fitEllipse(pts);
// 输出结果
std::cout << "椭圆中心:" << ellipse.center.x << "," << ellipse.center.y << std::endl;
std::cout << "椭圆长轴半径:" << ellipse.size.width / 2 << std::endl;
std::cout << "椭圆短轴半径:" << ellipse.size.height / 2 << std::endl;
std::cout << "椭圆旋转角度:" << ellipse.angle << std::endl;
return 0;
}
```
cv::fitCircle()函数用于拟合圆,该函数的输入参数为点集,输出参数为圆心坐标和半径。具体实现如下:
```cpp
#include <opencv2/opencv.hpp>
int main()
{
// 构造点集
std::vector<cv::Point2f> pts;
pts.push_back(cv::Point2f(50, 50));
pts.push_back(cv::Point2f(100, 100));
pts.push_back(cv::Point2f(150, 50));
// 拟合圆
cv::Point2f center;
float radius;
cv::minEnclosingCircle(pts, center, radius);
// 输出结果
std::cout << "圆心坐标:" << center.x << "," << center.y << std::endl;
std::cout << "半径:" << radius << std::endl;
return 0;
}
```
需要注意的是,cv::fitCircle()函数只能拟合凸点集,如果点集不是凸的,则需要使用cv::minEnclosingCircle()函数来拟合圆。
相关推荐
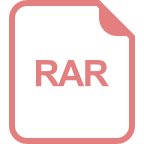













