1.查看当前路径下所有的子文件,输入已有的两个文件名,判断两个文件谁新,将新文件的所有内容写入到旧文件中,并计算出现在旧文件的大小 (使用shell脚本编写)【】
时间: 2024-02-06 16:10:49 浏览: 25
可以使用以下的 shell 脚本来实现:
```bash
#!/bin/bash
file1=$1
file2=$2
if [[ ! -f $file1 || ! -f $file2 ]]; then
echo "Error: Both files must exist"
exit 1
fi
if [[ $file1 -nt $file2 ]]; then
# file1 is newer than file2
cat $file1 > $file2
size=$(stat -c%s "$file2")
else
# file2 is newer than or equal to file1
cat $file2 > $file1
size=$(stat -c%s "$file1")
fi
echo "New size of $file1: $size bytes"
```
这个脚本接受两个文件名作为输入,并且首先检查这两个文件是否都存在。如果其中一个文件不存在,脚本将会输出错误信息并退出。
接下来,脚本会比较这两个文件的修改时间戳(使用 `-nt` 选项),以判断哪个文件更加新。如果 file1 比 file2 新,脚本会把 file1 中的内容写入到 file2 中,否则将 file2 中的内容写入到 file1 中。
最后,脚本会使用 `stat` 命令来获取旧文件的大小,并输出这个大小。
相关问题
请看两个js文件,Nodejs运行2.js发生错误 :
很抱歉,我无法看到你的文件,但我可以尝试帮助你找到错误。
首先,请确认您的代码是否正确。如果您确定代码没有问题,可以检查以下几点:
1. 检查文件名和路径是否正确。
2. 确保您在运行2.js之前已安装了所有必需的依赖项。
3. 检查您的代码是否需要使用特定版本的Node.js。
4. 尝试从终端窗口运行2.js,看看是否有任何错误消息输出。
5. 如果错误消息不明确,请尝试在代码中添加调试语句,以帮助您找到问题所在。
如果您仍然无法找到错误,请尝试使用调试工具,如Node.js自带的调试器或VS Code的调试功能。
用python写一段代码:文件夹下有多个json文件,和另一个文件夹下的json文件进行对比,删除文件名重复的json文件
以下是一个示例代码,用于比较两个文件夹中的JSON文件,并删除文件名重复的JSON文件:
```python
import os
# 指定两个文件夹的路径
folder1_path = "path/to/folder1" # 第一个文件夹的路径
folder2_path = "path/to/folder2" # 第二个文件夹的路径
# 获取两个文件夹中的所有JSON文件名
folder1_files = [f for f in os.listdir(folder1_path) if f.endswith(".json")]
folder2_files = [f for f in os.listdir(folder2_path) if f.endswith(".json")]
# 找出重复的文件名,并删除第一个文件夹中的重复文件
for file in folder1_files:
if file in folder2_files:
file_path = os.path.join(folder1_path, file)
os.remove(file_path)
print(f"已删除文件: {file}")
print("删除完成")
```
请将上述示例代码中的`folder1_path`和`folder2_path`替换为你实际的文件夹路径。代码首先使用`os.listdir()`函数获取两个文件夹中的所有JSON文件名,并存储在`folder1_files`和`folder2_files`列表中。然后,使用循环遍历`folder1_files`列表,如果文件名也在`folder2_files`列表中,则删除第一个文件夹中的重复文件。最后,输出删除完成的提示信息。请确保在运行代码前备份你的数据,以防不必要的数据丢失。
相关推荐
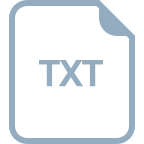
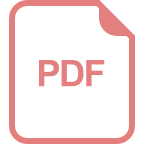
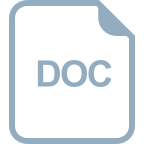












