libcurl下载文件
时间: 2024-10-31 19:08:12 浏览: 18
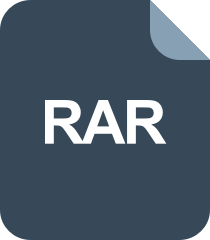
libcurl下载文件并显示进度

`libcurl` 是一个强大的开源库,用于从 URL 获取数据,支持多种协议如 HTTP、HTTPS、FTP 等。如果你想使用 libcurl 下载文件到本地,你可以利用它的 API 来编写 C++ 代码。以下是一个简单的示例,展示了如何使用 libcurl 下载文件:
```cpp
#include <iostream>
#include <curl/curl.h>
size_t write_data(void* ptr, size_t size, size_t nmemb, std::string* data) {
// 这个回调函数会被 libcurl 执行,当有数据可用时,将其写入到字符串中
data->append(static_cast<char*>(ptr), size * nmemb);
return size * nmemb;
}
int main() {
CURL* curl;
CURLcode res;
std::string file_path;
// 初始化 curl
curl_global_init(CURL_GLOBAL_DEFAULT);
// 创建 curl handle
curl = curl_easy_init();
if (!curl) {
std::cerr << "Could not initialize curl" << std::endl;
return 1;
}
// 设置要下载的 URL 和输出文件路径
file_path = "/path/to/downloaded/file.txt";
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com/file.txt");
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, write_data);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &file_path);
// 开始下载
res = curl_easy_perform(curl);
// 检查错误并清理
if (res != CURLE_OK) {
std::cerr << "Error downloading: " << curl_easy_strerror(res) << std::endl;
}
curl_easy_cleanup(curl);
// 清理全局资源
curl_global_cleanup();
return 0;
}
```
这个程序首先初始化了 `libcurl`,然后创建了一个 `CURL` 对象,设置要下载的 URL 和一个回调函数(`write_data`)用于接收下载的数据并将其写入到指定的文件中。然后调用 `curl_easy_perform()` 发起下载请求。如果下载成功,`main` 函数会返回 0,否则会打印错误信息。
阅读全文
相关推荐
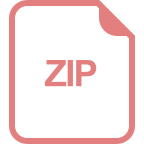
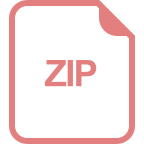

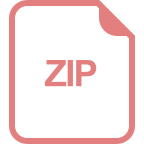
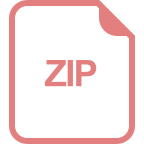


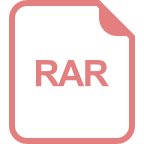
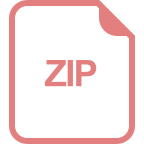
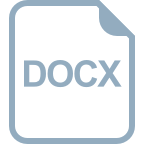
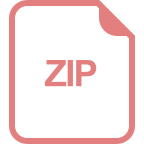
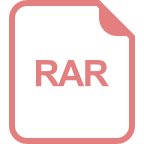
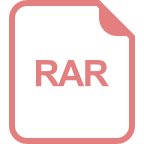





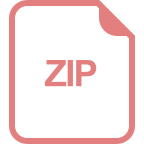