二维粒子滤波python
时间: 2023-10-31 09:07:39 浏览: 46
二维粒子滤波是一种基于蒙特卡罗方法的状态估计算法,常用于机器人自定位和目标跟踪等领域。在二维粒子滤波中,机器人的状态被表示为一个二维向量,包括机器人的位置和方向。粒子滤波的核心思想是通过一系列粒子来近似表示机器人的状态分布,然后根据测量数据和运动模型对粒子进行更新和重采样,从而实现对机器人状态的估计。
在Python中,可以使用numpy和matplotlib等库来实现二维粒子滤波算法。具体实现过程包括初始化粒子、运动模型、测量模型、重采样等步骤。其中,权值的计算是粒子滤波器的关键一步,它决定了一次迭代中哪些粒子能够存活。在每次迭代中,粒子的权值会根据测量数据和运动模型进行更新,然后根据权值进行重采样,从而得到下一次迭代的粒子集合。
相关问题
一维粒子滤波python代码
以下是一维粒子滤波的python代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
def motion_model(x, u):
# 一维运动模型
return x + u + np.random.normal(0, 1)
def sensor_model(x, z):
# 一维测量模型
return np.exp(-(x-z)**2/2) / np.sqrt(2*np.pi)
def resampling(particles, weights):
# 重采样
N = len(particles)
indexes = np.zeros(N, dtype=int)
cumulative_sum = np.cumsum(weights)
i, j = 0, 0
while i < N and j < N:
if np.random.rand() < cumulative_sum[j]:
indexes[i] = j
i += 1
else:
j += 1
return particles[indexes], np.ones(N) / N
def main():
# 初始化粒子
N = 100
particles = np.random.normal(0, 1, N)
weights = np.ones(N) / N
# 运动和测量模型
u = 1
z = 2
# 粒子滤波
for i in range(10):
# 运动模型
particles = motion_model(particles, u)
# 权重计算
weights *= sensor_model(particles, z)
weights /= np.sum(weights)
# 重采样
particles, weights = resampling(particles, weights)
# 结果可视化
plt.hist(particles, bins=20, weights=weights)
plt.show()
if __name__ == '__main__':
main()
```
在这个示例中,我们使用了一维的运动模型和测量模型,并且每次迭代都进行一次重采样。最后,我们将粒子的分布可视化为一个直方图。
二维粒子滤波matlab
二维粒子滤波是一种用于目标跟踪和状态估计的随机滤波方法,它基于粒子滤波算法,能够有效地处理非线性和非高斯性的系统。在Matlab中,我们可以使用内置的函数和工具箱来实现二维粒子滤波。
首先,我们需要定义系统模型和测量模型。系统模型描述了目标在状态转移过程中的动态特性,而测量模型描述了观测到的目标状态的特性。然后,我们需要初始化一组粒子,这些粒子会根据系统模型进行状态转移,同时根据测量模型进行更新。
在Matlab中,我们可以使用函数如particleFilter和resample来实现二维粒子滤波。particleFilter函数用于实现粒子的状态转移和更新过程,而resample函数用于根据粒子的权重进行重采样,以保持粒子的多样性和准确性。
此外,我们还可以利用Matlab中的图形化工具箱,如plot函数和animation函数,来实时显示粒子的分布和目标的轨迹,以帮助我们理解和调试滤波算法。
总之,在Matlab中实现二维粒子滤波需要明确系统模型和测量模型,合理初始化粒子集合,并利用内置函数和图形化工具箱来实现算法并进行可视化展示。通过这些步骤,我们可以轻松地在Matlab中实现和调试二维粒子滤波算法。
相关推荐
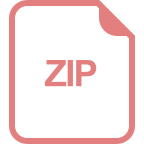












