自定义学生类:包含姓名,年龄,成绩 属性为私有成员变量,并生成无参,有参构造方法,生成get/set方法.创建5个学生放到 ArrayList中.使用迭代器获取每个学生信息.统计总分,平均分,最高分,最低分并输出。(实现思路如下:) ① 定义学生类生成类无参,有参构造方法,生成get/set方法. ② 定义ArrayList ③ 添加5个学生 ④ 获取迭代器 ⑤ 定义总分 ⑥ 定义最高分 ⑦ 定义最低分 ⑧ 循环判断是否有下一个学生 ⑨ 使用next方法获取学生对象 ⑩ 将这个学生对象的分数添加到总分 11 判断这个学生的分数是否大于最大分数 12 这个学生的分数大于最大分数.最大分数等于这个学生的分数 13 判断这个学生的分数是否大低最大分数 14 这个学生的分数小于最小分数.最小分数等于这个学生的分数 15 计算平均分(平均分 = 总分/人数) 16 打印总分,最高分,最低分
时间: 2024-04-21 08:22:20 浏览: 20
以下是实现上述功能的示例代码:
```java
import java.util.ArrayList;
import java.util.Iterator;
// 定义学生类
class Student {
private String name;
private int age;
private double score;
public Student() {
}
public Student(String name, int age, double score) {
this.name = name;
this.age = age;
this.score = score;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public double getScore() {
return score;
}
public void setScore(double score) {
this.score = score;
}
}
public class Main {
public static void main(String[] args) {
// 创建ArrayList并添加5个学生
ArrayList<Student> students = new ArrayList<>();
students.add(new Student("Alice", 18, 90));
students.add(new Student("Bob", 19, 85));
students.add(new Student("Charlie", 17, 92));
students.add(new Student("David", 20, 88));
students.add(new Student("Eve", 19, 95));
// 使用迭代器获取每个学生信息
Iterator<Student> iterator = students.iterator();
double totalScore = 0; // 总分
double maxScore = Double.MIN_VALUE; // 最高分
double minScore = Double.MAX_VALUE; // 最低分
while (iterator.hasNext()) {
Student student = iterator.next();
totalScore += student.getScore();
if (student.getScore() > maxScore) {
maxScore = student.getScore();
}
if (student.getScore() < minScore) {
minScore = student.getScore();
}
}
int numOfStudents = students.size();
double averageScore = totalScore / numOfStudents; // 平均分
// 输出统计结果
System.out.println("总分:" + totalScore);
System.out.println("平均分:" + averageScore);
System.out.println("最高分:" + maxScore);
System.out.println("最低分:" + minScore);
}
}
```
请注意,这只是一个实现示例,你可以根据自己的需求进行修改和扩展。
相关推荐
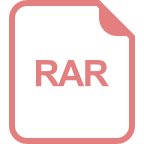
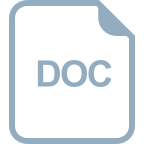
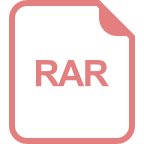












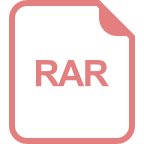
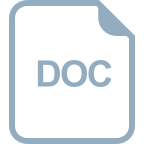
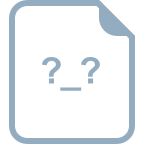
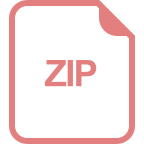