编写一个程序 采用顺序表 存储一元多项式,并实现两个多项式相加运算,要求: (1)创建存储多项式的有序表(按无序输入)ha和 hb;(2)求ha和hb相加产生多项式hc。(3)输出多项式ha、hb和hc;
时间: 2024-11-29 12:37:05 浏览: 20
为了编写一个顺序表结构用于存储一元多项式,并实现在Python中完成两个多项式相加的功能,你可以按照以下步骤进行:
1. **定义多项式结构**:
首先,我们可以定义一个`Term`类表示多项式的单个项(系数乘以变量的幂次),以及一个`Polynomial`类,它包含一个有序列表来存储多项式的各个项。
```python
class Term:
def __init__(self, coefficient, exponent):
self.coefficient = coefficient
self.exponent = exponent
class Polynomial:
def __init__(self):
self.terms = []
def add_term(self, term):
self.terms.append(term)
def __str__(self):
# 输出多项式字符串格式
result = ""
for term in self.terms:
if term.coefficient != 0:
result += f"{term.coefficient}x^{{{term.exponent}}}, "
return result.strip() + " (if x=1)"
```
2. **输入并构建多项式**:
创建两个多项式实例`ha`和`hb`,通过循环接收用户输入(系数和指数),并将它们添加到对应的多项式中。
```python
def build_polynomial(name):
polynomial = Polynomial()
while True:
try:
coefficient = float(input(f"请输入{name}的系数({name}_coefficient): "))
exponent = int(input(f"请输入{name}的指数({name}_exponent): "))
term = Term(coefficient, exponent)
polynomial.add_term(term)
except ValueError:
break
return polynomial
ha = build_polynomial("ha")
hb = build_polynomial("hb")
```
3. **相加多项式**:
定义一个`add`方法,遍历两个多项式的所有项,将系数累加,然后合并到`hc`中。
```python
def add_poly(ha, hb):
hc = Polynomial()
for term_ha, term_hb in zip(ha.terms, hb.terms):
new_coeff = term_ha.coefficient + term_hb.coefficient
new_exponent = max(term_ha.exponent, term_hb.exponent)
new_term = Term(new_coeff, new_exponent)
hc.add_term(new_term)
return hc
```
4. **输出结果**:
最后,打印出原始多项式`ha`、`hb`以及它们的和`hc`。
```python
def print_polynomials(ha, hb, hc):
print(f"{ha}\n{hb}\n他们的和是:")
print(hc)
print_polynomials(ha, hb, add_poly(ha, hb))
```
阅读全文
相关推荐
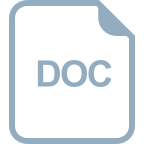
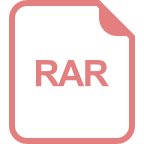















