创建一个包含多个结构体的容器
时间: 2024-02-26 09:59:20 浏览: 22
可以使用 C++ 中的 STL 容器来存储多个结构体。其中,常用的有 vector、array 和 list 等。以 vector 为例,假设有以下两个结构体:
```
struct Person {
string name;
int age;
};
struct Book {
string title;
string author;
double price;
};
```
我们可以创建一个 vector 容器,用来存储多个 Person 和 Book 结构体:
```
vector<Person> people;
vector<Book> books;
```
然后,我们可以向容器中添加元素,例如:
```
Person p1 = {"Alice", 25};
Person p2 = {"Bob", 30};
people.push_back(p1);
people.push_back(p2);
Book b1 = {"The Catcher in the Rye", "J.D. Salinger", 9.99};
Book b2 = {"To Kill a Mockingbird", "Harper Lee", 8.99};
books.push_back(b1);
books.push_back(b2);
```
我们也可以通过迭代器遍历容器中的元素:
```
for (auto it = people.begin(); it != people.end(); ++it) {
cout << it->name << ", " << it->age << endl;
}
for (auto it = books.begin(); it != books.end(); ++it) {
cout << it->title << ", " << it->author << ", " << it->price << endl;
}
```
相关推荐
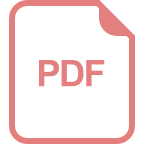














