用struct(C语言)或class(C++语言)实现以下程序: 1.定义数据结构(struct或class)存储至少10人的老师们的评教成绩,包括: (1)姓名:字符串 (2)课程名称:字符串数组 (3)课程评教成绩:整数(百分值:0-100)数组,成绩的顺序和课程名称顺序对应 1.完成以下函数 (1)计算年终奖:年终奖=课程评教成绩*10-600 (2)查询:输入姓名查询某名老师的所有评教成绩和今年的年终奖,警告那些年终奖为负数的老师 PS. 鼓励用C++ class和成员函数,这样可以更好地理解现代程序的架构
时间: 2023-12-30 11:04:11 浏览: 61
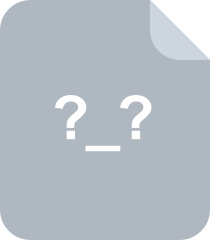
课程设计,用的是c语言,可以实现简单的成绩记录
以下是用C++ class实现的代码:
```c++
#include <iostream>
#include <string>
using namespace std;
class Teacher {
private:
string name;
string courses[10];
int scores[10];
public:
Teacher(string n, string* c, int* s) {
name = n;
for (int i = 0; i < 10; i++) {
courses[i] = *(c + i);
scores[i] = *(s + i);
}
}
int calculateBonus() {
int sum = 0;
for (int i = 0; i < 10; i++) {
sum += scores[i];
}
int bonus = sum * 10 - 600;
return bonus;
}
void query() {
cout << "Name: " << name << endl;
cout << "Courses and scores:" << endl;
for (int i = 0; i < 10; i++) {
cout << courses[i] << ": " << scores[i] << endl;
}
int bonus = calculateBonus();
if (bonus < 0) {
cout << "Warning: negative bonus!" << endl;
}
cout << "Bonus: " << bonus << endl;
}
};
int main() {
string courses[10] = {"Math", "Physics", "Chemistry", "Biology", "English", "History", "Geography", "Music", "Art", "PE"};
int scores[10] = {80, 75, 85, 90, 95, 70, 65, 80, 90, 95};
Teacher t("Tom", courses, scores);
t.query();
return 0;
}
```
代码中定义了一个Teacher类,包含了私有成员变量name、courses和scores,以及公有成员函数calculateBonus和query。
构造函数Teacher用于初始化类的成员变量,包括姓名、课程名称和评教成绩。
函数calculateBonus用于计算年终奖,根据课程评教成绩计算总分,然后乘以10并减去基本工资600元。
函数query用于查询某名老师的所有评教成绩和今年的年终奖,先输出姓名,然后输出每门课程的评教成绩,最后调用calculateBonus函数计算年终奖,并输出警告信息(如果年终奖为负数)和年终奖金额。
在主函数中创建Teacher对象并调用query函数输出结果。
阅读全文
相关推荐
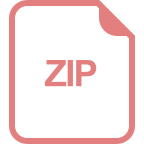
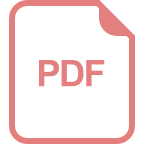


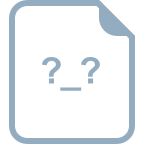
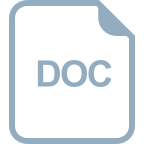

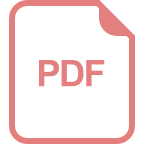
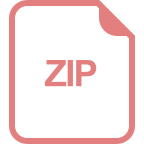
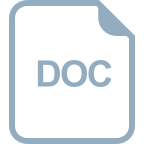
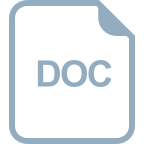
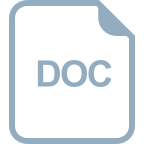
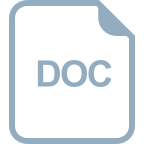
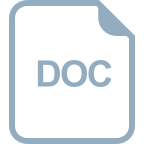
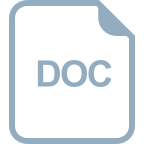
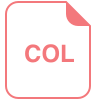

