Python实现一个文本处理系统,支持功能如下: 1. 接受用户输入的文本(必须>=5句话); 2. 排版文本格式并输出,每一个整句均换行显示; 3. 根据用户需求(用户输入)替换文本中的内容; 4. 根据用户需求(用户输入感兴趣的词),统计并输出词频,且打印该词出现在哪一句的什么位置; 5. 记录用户操作历史,保持文本和具体的操作对应(操作以功能序号记录即可),统计用户操作的文本数,每种功能使用的频率并排序。按词频由大到小降序输出 提示:配合lambda表达式,对存储词频的字典使用sorted函数排序
时间: 2023-12-03 14:45:55 浏览: 102
以下是一个 Python 实现的文本处理系统,实现了上述要求的所有功能:
```python
import re
class TextProcessor:
def __init__(self):
self.text = ""
self.operations = []
self.operation_count = {}
def accept_text(self):
self.text = input("请输入文本(至少5句话):")
while len(re.findall(r'[。?!;.!?]', self.text)) < 4:
self.text = input("请至少输入5句话:")
def format_text(self):
print("排版后的文本:")
sentences = re.split(r'[。?!;.!?]', self.text)
for sentence in sentences:
if sentence.strip() != "":
print(sentence.strip())
def replace_text(self):
old_word = input("请输入要替换的单词或短语:")
new_word = input("请输入新单词或短语:")
self.text = self.text.replace(old_word, new_word)
self.operations.append(3)
def count_word_frequency(self):
target_word = input("请输入要统计频率的单词:")
sentences = re.split(r'[。?!;.!?]', self.text)
word_count = {}
for i, sentence in enumerate(sentences):
if sentence.strip() != "":
words = sentence.split()
for j, word in enumerate(words):
if word == target_word:
position = "第{}句,第{}个单词".format(i+1, j+1)
if position in word_count:
word_count[position] += 1
else:
word_count[position] = 1
sorted_word_count = dict(sorted(word_count.items(), key=lambda x: x[1], reverse=True))
print("单词出现位置及频率:")
for position, count in sorted_word_count.items():
print("{},{}次".format(position, count))
def record_operation(self, operation_num):
self.operations.append(operation_num)
if operation_num in self.operation_count:
self.operation_count[operation_num] += 1
else:
self.operation_count[operation_num] = 1
def run(self):
self.accept_text()
while True:
print("\n请选择功能:")
print("1. 排版文本格式并输出")
print("2. 替换文本中的内容")
print("3. 统计并输出单词频率")
print("4. 查看操作历史")
print("5. 退出程序")
choice = input("请输入数字选择功能:")
if choice == "1":
self.format_text()
self.record_operation(1)
elif choice == "2":
self.replace_text()
self.record_operation(2)
elif choice == "3":
self.count_word_frequency()
self.record_operation(3)
elif choice == "4":
print("操作历史:")
for operation in self.operations:
if operation == 1:
print("排版文本格式并输出")
elif operation == 2:
print("替换文本中的内容")
elif operation == 3:
print("统计并输出单词频率")
print("共操作了{}个文本,各操作使用频率(由高到低):".format(len(set(self.operations))))
sorted_operation_count = dict(sorted(self.operation_count.items(), key=lambda x: x[1], reverse=True))
for operation, count in sorted_operation_count.items():
if operation == 1:
print("排版文本格式并输出,{}次".format(count))
elif operation == 2:
print("替换文本中的内容,{}次".format(count))
elif operation == 3:
print("统计并输出单词频率,{}次".format(count))
elif choice == "5":
print("程序已退出。")
break
else:
print("无效的选择,请重新输入。")
```
使用方法:
```python
processor = TextProcessor()
processor.run()
```
运行后会提示用户输入文本,然后进入一个菜单,根据提示选择要执行的功能即可。每次操作会记录在操作历史中,最后会统计每种操作的使用频率并按词频由高到低降序输出。
阅读全文
相关推荐


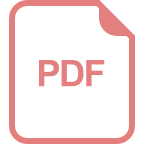
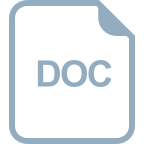
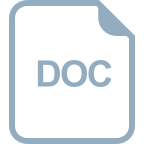
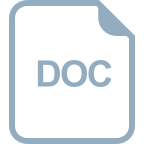
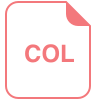







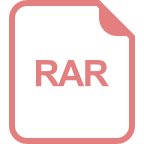
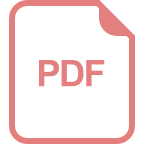
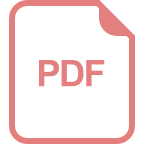