ProcessPoolExecutor的用法
时间: 2024-10-01 13:12:29 浏览: 19
`ProcessPoolExecutor`是Python `concurrent.futures`模块中的一个组件,它允许你在多进程中并行地执行任务。它的基本用法包括:
1. **创建进程池**:
```python
from concurrent.futures import ProcessPoolExecutor
with ProcessPoolExecutor(max_workers=4) as executor:
# max_workers指定并发处理的最大进程数
```
2. **提交任务**:
```python
def worker_func(data):
# 这里是你想在新进程中运行的函数
...
futures = [executor.submit(worker_func, i) for i in range(10)]
```
这里通过`submit()`方法将任务(这里是`worker_func`)及其参数提交给进程池。
3. **获取结果**:
```python
for future in futures:
result = future.result() # 等待任务完成并获取结果
print(result)
```
如果任务执行成功,`result()`会返回任务的结果;如果出错,将会抛出异常。
相关问题
processpoolexecutor
ProcessPoolExecutor是Python中concurrent.futures库中的一个类,它允许在多个进程中并行执行计算。它使用了进程池来管理进程,可以通过submit()方法提交要执行的函数和参数,并返回一个Future对象来表示该计算。可以使用as_completed()或者wait()来获取结果。
举例说明 ProcessPoolExecutor
ProcessPoolExecutor 是一个 Python 标准库中的并发编程模块,它提供了一个方便的方式来并行地执行 Python 函数。它使用进程池来执行函数,每个进程都运行在其自己的操作系统进程中。
举个例子,假设我们有一个函数 `calculate_square()`,它接受一个整数并返回该整数的平方。我们可以使用 ProcessPoolExecutor 来并行计算多个整数的平方:
```python
from concurrent.futures import ProcessPoolExecutor
def calculate_square(x):
return x ** 2
if __name__ == '__main__':
with ProcessPoolExecutor() as executor:
results = executor.map(calculate_square, [1, 2, 3, 4, 5])
print(list(results))
```
在这个例子中,我们使用了 `with` 语句来创建一个 ProcessPoolExecutor 对象,并使用 `map()` 方法来并行计算输入列表中每个整数的平方。最后,我们将结果转换为列表并打印出来。
由于 ProcessPoolExecutor 使用进程池,因此它可以利用多个 CPU 核心来并行执行函数,从而提高程序的性能。
阅读全文
相关推荐
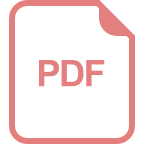
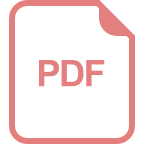
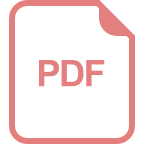

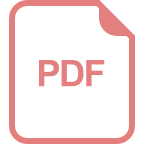
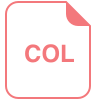









