module accumulator(input clk, reset, input [15:0] in, output reg [15:0] out); reg [15:0] sum; wire [15:0] carry; assign carry[0] = 1'b0; genvar i; generate for (i = 1; i < 16; i = i + 1) begin : adder full_adder adder(clk, reset, in[i], sum[i-1], carry[i-1], sum[i], carry[i]); end endgenerate always @(posedge clk) begin if (reset) begin sum <= 16'h0000; out <= 16'h0000; end else begin sum <= sum + in; out <= sum; end end endmodule module full_adder(input clk, reset, input a, b, c_in, output reg s, c_out); always @(posedge clk) begin if (reset) begin s <= 1'b0; c_out <= 1'b0; end else begin s <= a ^ b ^ c_in; c_out <= (a & b) | (a & c_in) | (b & c_in); end end endmodule为以上代码编写测试激励
时间: 2024-01-21 19:04:30 浏览: 139
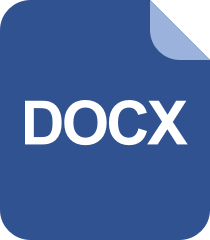
索引0-C--问题--已答2
以下是一个可能的测试激励代码:
```
`timescale 1ns / 1ps
module accumulator_tb;
// Inputs
reg clk;
reg reset;
reg [15:0] in;
// Outputs
wire [15:0] out;
// Instantiate the Unit Under Test (UUT)
accumulator uut (
.clk(clk),
.reset(reset),
.in(in),
.out(out)
);
initial begin
// Initialize inputs
clk = 0;
reset = 1;
in = 16'h0000;
// Wait for 10 clock cycles
#10;
// Deassert reset
reset = 0;
// Test case 1: Add 1 to accumulator
in = 16'h0001;
#10;
if (out !== 16'h0001) $display("Test case 1 failed: Expected out = 16'h0001, Actual out = %h", out);
// Test case 2: Add 2 to accumulator
in = 16'h0002;
#10;
if (out !== 16'h0003) $display("Test case 2 failed: Expected out = 16'h0003, Actual out = %h", out);
// Test case 3: Add -1 to accumulator
in = 16'hFFFF;
#10;
if (out !== 16'h0002) $display("Test case 3 failed: Expected out = 16'h0002, Actual out = %h", out);
// Test case 4: Add 0 to accumulator
in = 16'h0000;
#10;
if (out !== 16'h0002) $display("Test case 4 failed: Expected out = 16'h0002, Actual out = %h", out);
$display("Testing completed successfully!");
$finish;
end
// Toggle clock every 5 ns
always #5 clk <= ~clk;
endmodule
```
这个测试激励会对累加器进行四个测试,分别是:
1. 将1加入累加器,期望输出为1
2. 将2加入累加器,期望输出为3
3. 将-1加入累加器,期望输出为2
4. 将0加入累加器,期望输出为2
测试激励会在每个测试之间等待10个时钟周期,同时会在仿真结束时输出测试结果。注意,测试中并没有对溢出情况进行测试。
阅读全文
相关推荐
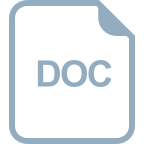
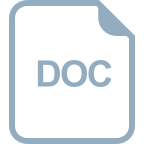
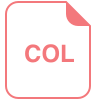
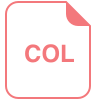

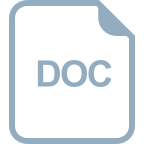
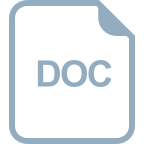
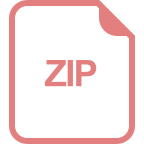
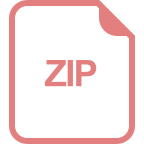
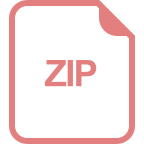
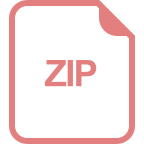
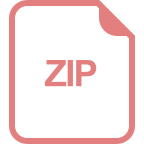