创建函数模板 1.创建 Student (数据成员 string name , int grade )类及所需函数。 2.求出集合中的最大值,支持 int , double , char , Student 等类型的数据。 3.排序方法,支持 int , double , char , Student (按成绩排序)等类型的数据。 4.中位数指的是一组数,如果按照大小排序排好后最中间的那个数的值, 如果有偶数个元素,那么就是最中间两个数的平均数。 求出集合中的中位数,支持 int , double , Student 集合中成绩的中位数。
时间: 2024-01-22 07:19:27 浏览: 79
以下是创建的函数模板:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
class Student {
public:
string name;
int grade;
bool operator<(const Student& rhs) const {
return grade < rhs.grade;
}
};
template <typename T>
T max_in_vector(const vector<T>& v) {
T max_val = v[0];
for (size_t i = 1; i < v.size(); i++) {
if (max_val < v[i]) max_val = v[i];
}
return max_val;
}
template <typename T>
void sort_vector(vector<T>& v) {
sort(v.begin(), v.end());
}
template <>
void sort_vector<Student>(vector<Student>& v) {
sort(v.begin(), v.end(), [](const Student& a, const Student& b) {
return a.grade < b.grade;
});
}
template <typename T>
T median(const vector<T>& v) {
size_t n = v.size() / 2;
vector<T> v_copy = v;
sort_vector(v_copy);
if (v.size() % 2 == 0) {
return (v_copy[n-1] + v_copy[n]) / 2;
}
else {
return v_copy[n];
}
}
int main() {
vector<int> v1 = {3, 6, 2, 7, 1, 9};
cout << "Max value in v1: " << max_in_vector(v1) << endl;
vector<double> v2 = {3.2, 6.7, 2.1, 7.8, 1.9, 9.4};
cout << "Max value in v2: " << max_in_vector(v2) << endl;
vector<char> v3 = {'c', 'g', 'a', 'f', 'b', 'd'};
cout << "Max value in v3: " << max_in_vector(v3) << endl;
vector<Student> v4 = {
{"Alice", 85},
{"Bob", 92},
{"Charlie", 77},
{"David", 80},
{"Eva", 88}
};
cout << "Max value in v4: " << max_in_vector(v4).grade << endl;
vector<int> v5 = {3, 6, 2, 7, 1, 9};
sort_vector(v5);
for (auto x : v5) cout << x << " ";
cout << endl;
vector<double> v6 = {3.2, 6.7, 2.1, 7.8, 1.9, 9.4};
sort_vector(v6);
for (auto x : v6) cout << x << " ";
cout << endl;
vector<char> v7 = {'c', 'g', 'a', 'f', 'b', 'd'};
sort_vector(v7);
for (auto x : v7) cout << x << " ";
cout << endl;
vector<Student> v8 = {
{"Alice", 85},
{"Bob", 92},
{"Charlie", 77},
{"David", 80},
{"Eva", 88}
};
sort_vector(v8);
for (auto x : v8) cout << x.name << " " << x.grade << endl;
vector<int> v9 = {3, 6, 2, 7, 1, 9};
cout << "Median of v9: " << median(v9) << endl;
vector<double> v10 = {3.2, 6.7, 2.1, 7.8, 1.9, 9.4};
cout << "Median of v10: " << median(v10) << endl;
vector<Student> v11 = {
{"Alice", 85},
{"Bob", 92},
{"Charlie", 77},
{"David", 80},
{"Eva", 88}
};
cout << "Median of v11: " << median(v11).grade << endl;
return 0;
}
```
其中,`max_in_vector` 函数可以求出任意类型(`int`、`double`、`char`、`Student`)的向量中的最大值;`sort_vector` 函数可以对任意类型的向量进行排序,其中对 `Student` 类型排序时按照成绩从小到大排序;`median` 函数可以求出任意类型(`int`、`double`、`Student`)的向量中的中位数,其中对 `Student` 类型的向量求中位数时按照成绩从小到大排序。
阅读全文
相关推荐
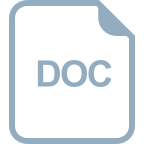
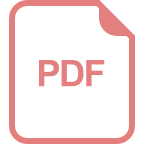
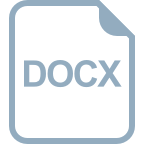













