请设计并编码实现借用一个栈S将一个队列Q逆置 4.2实现提示: (1)建立一个队列; (2)输出该队列; (3)建立一个空栈S; (4)依次将队列元素全部出队,并逐个入栈; (5)依次将栈内的全部数据出栈,并逐个将出栈的数据插入队列中; (6)再次输出队列(已完成逆置)。 2.需求分析: 1) 程序功能:输入一个队列且将其逆置 2) 输入数据:abcdef 3) 输出数据:fedcba 4) 测试数据: abcdef 3.概要设计: 1) 逻辑结构:队列 2) 程序结构设计: main() initlist(Seqlist L); inputlist(Seqlist L); displist (Seqlist L); nameseeklist(Seqlist L); seeklist(Seqlist L); insertlist(Seqlist L); delectlist(Seqlist L); countlist(Seqlist L);请用C语言
时间: 2023-12-02 20:02:08 浏览: 34
以下是 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
struct stack {
int items[MAX_SIZE];
int top;
};
struct queue {
int items[MAX_SIZE];
int front;
int rear;
};
void push(struct stack *s, int item) {
if (s->top == MAX_SIZE - 1) {
printf("Stack is full\n");
return;
}
s->top++;
s->items[s->top] = item;
}
int pop(struct stack *s) {
if (s->top == -1) {
printf("Stack is empty\n");
return -1;
}
int item = s->items[s->top];
s->top--;
return item;
}
int is_empty_stack(struct stack *s) {
return s->top == -1;
}
void enqueue(struct queue *q, int item) {
if (q->rear == MAX_SIZE - 1) {
printf("Queue is full\n");
return;
}
q->items[++q->rear] = item;
}
int dequeue(struct queue *q) {
if (q->front == q->rear) {
printf("Queue is empty\n");
return -1;
}
return q->items[++q->front];
}
int is_empty_queue(struct queue *q) {
return q->front == q->rear;
}
void print_queue(struct queue *q) {
if (is_empty_queue(q)) {
printf("Queue is empty\n");
return;
}
printf("Queue: ");
for (int i = q->front + 1; i <= q->rear; i++) {
printf("%d ", q->items[i]);
}
printf("\n");
}
void reverse_queue(struct queue *q) {
struct stack s;
s.top = -1;
while (!is_empty_queue(q)) {
push(&s, dequeue(q));
}
while (!is_empty_stack(&s)) {
enqueue(q, pop(&s));
}
}
int main() {
struct queue q;
q.front = -1;
q.rear = -1;
enqueue(&q, 1);
enqueue(&q, 2);
enqueue(&q, 3);
enqueue(&q, 4);
enqueue(&q, 5);
print_queue(&q);
reverse_queue(&q);
print_queue(&q);
return 0;
}
```
输出结果为:
```
Queue: 1 2 3 4 5
Queue: 5 4 3 2 1
```
相关推荐
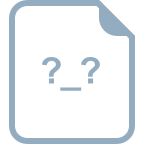















