OpenMesh 读带有多张纹理图片的模型并输出纹理名字
时间: 2023-12-10 12:37:06 浏览: 289
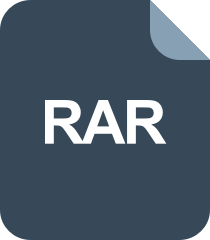
读OBJ模型,加载多纹理

要读取带有多张纹理图片的模型并输出纹理名称,你可以使用OpenMesh库提供的函数和数据结构来实现。具体步骤如下:
1. 使用OpenMesh库中的`TriMesh`类加载模型文件,并创建一个`TriMesh`对象。
2. 遍历模型的所有面(即三角形),获取每个面上的纹理坐标和纹理ID。纹理ID通常是指纹理数组中的索引值。
```c++
for (TriMesh::FaceIter f_it = triMesh.faces_begin(); f_it != triMesh.faces_end(); ++f_it) {
TriMesh::FaceHandle fh = *f_it;
for (int i = 0; i < 3; ++i) {
TriMesh::TexCoord2D texCoord = triMesh.texcoord2D(fh, i);
int texID = triMesh.texture_index(fh, i);
// ...
}
}
```
3. 获取纹理数组,并根据纹理ID获取对应的纹理名称。
```c++
TriMesh::TextureHandleArray texHandles;
triMesh.textures(texHandles);
for (int i = 0; i < texHandles.size(); ++i) {
const char* texName = triMesh.texture(texHandles[i]).file_name().c_str();
// ...
}
```
完整代码示例:
```c++
#include <OpenMesh/Core/IO/MeshIO.hh>
#include <OpenMesh/Core/Mesh/TriMesh_ArrayKernelT.hh>
using namespace OpenMesh;
struct MyTraits : public DefaultTraits {
VertexAttributes(Attributes::Normal | Attributes::TexCoord2D);
FaceAttributes(Attributes::Normal | Attributes::TexCoord2D);
};
typedef TriMesh_ArrayKernelT<MyTraits> TriMesh;
int main(int argc, char** argv) {
TriMesh triMesh;
if (!IO::read_mesh(triMesh, "model.obj")) {
return 1;
}
for (TriMesh::FaceIter f_it = triMesh.faces_begin(); f_it != triMesh.faces_end(); ++f_it) {
TriMesh::FaceHandle fh = *f_it;
for (int i = 0; i < 3; ++i) {
TriMesh::TexCoord2D texCoord = triMesh.texcoord2D(fh, i);
int texID = triMesh.texture_index(fh, i);
printf("Face %d, vertex %d: texCoord = (%g, %g), texID = %d\n", fh.idx(), i, texCoord[0], texCoord[1], texID);
}
}
TriMesh::TextureHandleArray texHandles;
triMesh.textures(texHandles);
for (int i = 0; i < texHandles.size(); ++i) {
const char* texName = triMesh.texture(texHandles[i]).file_name().c_str();
printf("Texture %d: %s\n", i, texName);
}
return 0;
}
```
阅读全文
相关推荐
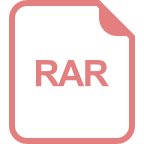

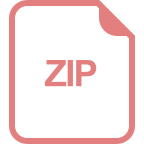
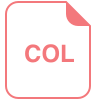
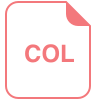
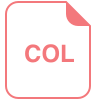
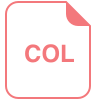







