经验模态分解代码可以在cuda上运行的
时间: 2024-06-11 20:10:50 浏览: 85
经验模态分解(Empirical Mode Decomposition,EMD)是一种信号处理方法,用于将非线性和非平稳信号分解成有限个本征模态函数(Intrinsic Mode Functions,IMF)的加和形式。EMD算法是一种迭代算法,每次迭代都会生成一个IMF,直到剩下一个残差信号。由于EMD算法中的迭代过程非常复杂,因此实现EMD算法的代码通常比较复杂。
在GPU上实现EMD算法可以大大提高计算速度。CUDA是NVIDIA公司开发的一种并行计算平台和编程模型,可以用于实现GPU加速的计算。以下是用CUDA实现EMD算法的代码示例:
```c++
#include <cuda_runtime.h>
#include <device_launch_parameters.h>
#include <math.h>
#include <stdio.h>
#define BLOCK_SIZE 1024
__global__ void emd_kernel(float *input, float *output, int num_points) {
int tid = threadIdx.x + blockIdx.x * blockDim.x;
// TODO: Implement EMD algorithm using CUDA
}
void emd(float *input, float *output, int num_points) {
int num_blocks = ceil(num_points / BLOCK_SIZE);
float *d_input, *d_output;
cudaMalloc((void **)&d_input, num_points * sizeof(float));
cudaMalloc((void **)&d_output, num_points * sizeof(float));
cudaMemcpy(d_input, input, num_points * sizeof(float), cudaMemcpyHostToDevice);
emd_kernel<<<num_blocks, BLOCK_SIZE>>>(d_input, d_output, num_points);
cudaMemcpy(output, d_output, num_points * sizeof(float), cudaMemcpyDeviceToHost);
cudaFree(d_input);
cudaFree(d_output);
}
int main() {
int num_points = 1000;
float *input = (float *)malloc(num_points * sizeof(float));
float *output = (float *)malloc(num_points * sizeof(float));
// TODO: Initialize input array
emd(input, output, num_points);
// TODO: Process output array
free(input);
free(output);
return 0;
}
```
在上面的代码中,emd_kernel函数是EMD算法的CUDA实现。该函数将输入信号分解成IMF,并将结果保存在输出数组中。在主函数中,我们首先初始化输入数组,然后调用emd函数对其进行分解,最后处理输出数组。
需要注意的是,由于EMD算法中的迭代过程非常复杂,因此在实现EMD算法的CUDA代码时需要仔细考虑算法的逻辑和数据传输的方式,以确保最终结果的正确性。
阅读全文
相关推荐
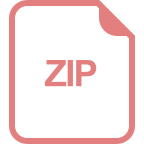
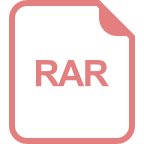
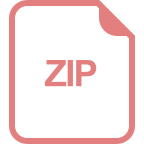
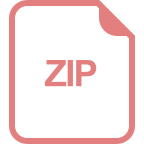
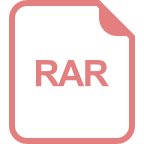
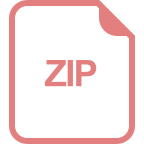
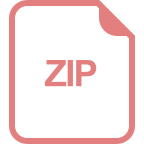
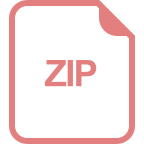
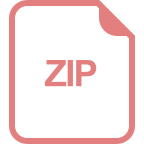
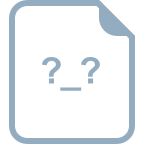
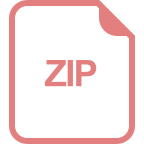
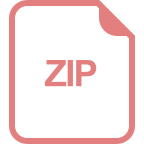
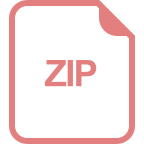
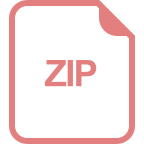
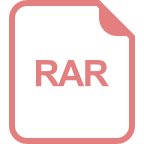
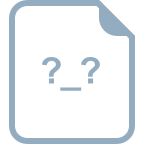
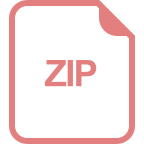