解释下面代码 #ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include <QKeyEvent> #include <QPushButton> #include <QPainter> #include <QTime> #include <QTimer> #include <QMouseEvent> #include <QDebug> //用来 Debug #include <QMessageBox>//自定义消息提示框 #include <QSettings> namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = nullptr); ~MainWindow(); //绘制格子 void paintEvent(QPaintEvent *); //键盘控制函数 void keyPressEvent(QKeyEvent *event); //移动函数 void PressUp(); void PressDown(); void PressLeft(); void PressRight(); //生成随机数2 void myRand(); //按钮指针 QPushButton *button_start; QPushButton *button_quit; QPushButton *button_rule; QPushButton *button_difficultysimple; QPushButton *button_difficulty; QPushButton *button_difficultyhell; QSettings settings; //定义格子 int s[4][4]; //分数 int score=0; int highScore = 0; //判断游戏的状态 bool state; struct Ns{ int i; int j; }; //槽函数——初始化 public slots: void slotStart_start(); int slotStart_simple(); int slotStart_difficulty(); int slotStart_hell(); void Message(); void closeEvent(); private: Ui::MainWindow *ui; }; #endif // MAINWINDOW_H
时间: 2024-04-04 10:34:46 浏览: 20
这是一个 C++ 的头文件,用于定义一个名为 MainWindow 的类。该类继承自 QMainWindow 类,包含了一些成员函数和成员变量,用于实现一个简单的游戏界面和游戏逻辑。其中包括绘制游戏界面的函数 paintEvent、键盘控制函数 keyPressEvent、移动函数 PressUp、PressDown、PressLeft、PressRight、生成随机数的函数 myRand、以及一些按钮指针和一些变量用于记录分数和游戏状态等。此外,还包括了一些 Qt 库的头文件和一些用于 Debug 的库,以及一个自定义的命名空间 Ui。头文件中还包含了一些预处理指令,用于避免头文件的重复引用。具体来说,#ifndef MAINWINDOW_H、#define MAINWINDOW_H、#endif // MAINWINDOW_H 用于定义头文件的保护机制,防止头文件被重复引用。
相关问题
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include <QKeyEvent> #include <QPushButton> #include <QPainter> #include <QTime> #include <QDebug> //用来 Debug #include <QMessageBox>//自定义消息提示框 namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = nullptr); ~MainWindow(); void paintEvent(QPaintEvent *); void keyPressEvent(QKeyEvent *event); void PressUp(); void PressDown(); void PressLeft(); void PressRight(); void myRand(); QPushButton *button; int s[4][4]; int score=0; bool state; struct Ns{ int i; int j; }; public slots: void slotStart(); private: Ui::MainWindow *ui; }; #endif // MAINWINDOW_H
这是一个Qt的头文件,用来定义MainWindow这个类。其中包含了一些Qt的类和命名空间。具体解释如下:
- #ifndef MAINWINDOW_H #define MAINWINDOW_H
这两行是用来防止头文件重复包含的宏定义,避免重复定义。如果已经定义了MAINWINDOW_H,则不会再次定义。
- #include <QMainWindow> #include <QKeyEvent> #include <QPushButton> #include <QPainter> #include <QTime> #include <QDebug> #include <QMessageBox>
这些是Qt的一些头文件,用来包含Qt的一些类和函数。例如,QMainWindow是Qt中的主窗口类,QKeyEvent用来处理键盘事件,QPushButton是按钮类,QPainter是绘图类,QTime是时间类,QDebug是用于调试的类,QMessageBox是自定义消息提示框。
- namespace Ui { class MainWindow; }
这是一个命名空间,用来定义MainWindow这个类。
- class MainWindow : public QMainWindow
这是一个类的定义,继承自QMainWindow类。在这个类中,我们可以定义变量、函数和槽函数。
- public:
这是一个访问限制符,表示接下来的成员函数和变量都是公有的,可以在其他类中访问。
- explicit MainWindow(QWidget *parent = nullptr);
这是MainWindow类的构造函数,用来初始化对象。
- ~MainWindow();
这是MainWindow类的析构函数,用来销毁对象。
- void paintEvent(QPaintEvent *);
这是一个虚函数,用来绘制窗口。
- void keyPressEvent(QKeyEvent *event);
这是一个函数,用来处理键盘按下事件。
- void PressUp(); void PressDown(); void PressLeft(); void PressRight(); void myRand();
这是一些函数,用来实现2048游戏的逻辑。PressUp函数表示向上移动方块,PressDown函数表示向下移动方块,PressLeft函数表示向左移动方块,PressRight函数表示向右移动方块,myRand函数表示生成新的方块。
- QPushButton *button;
这是一个指向QPushButton对象的指针。
- int s[4][4]; int score=0; bool state;
这是一些变量,用来存储2048游戏的状态和得分。
- struct Ns{ int i; int j; };
这是一个结构体,用来存储方块的位置。
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> namespace Ui { class MainWindow; } class MainWindow : public QMainWindow { Q_OBJECT public: explicit MainWindow(QWidget *parent = 0); ~MainWindow(); private: Ui::MainWindow *ui; }; #endif // MAINWINDOW_H对上面代码的每一行都进行详细的注释
```
#ifndef MAINWINDOW_H // 如果没有定义 MAINWINDOW_H 的宏常量,就执行下面的代码
#define MAINWINDOW_H // 定义 MAINWINDOW_H 的宏常量,防止头文件重复包含
#include <QMainWindow> // 包含 Qt 框架提供的 QMainWindow 类
namespace Ui { // 声明一个名为 Ui 的命名空间
class MainWindow; // 声明一个 MainWindow 类
}
class MainWindow : public QMainWindow { // 定义 MainWindow 类,继承 QMainWindow 类
Q_OBJECT // 用于使用信号和槽机制
public:
explicit MainWindow(QWidget *parent = 0); // 构造函数,explicit 用于防止隐式转换
~MainWindow(); // 析构函数
private:
Ui::MainWindow *ui; // 定义一个名为 ui 的指针,类型为 Ui::MainWindow,用于访问 MainWindow 的界面元素
};
#endif // MAINWINDOW_H // 结束头文件的定义,防止头文件重复包含
```
以上就是 `mainwindow.h` 文件的每一行注释。主要作用是定义了一个名为 MainWindow 的类,继承了 QMainWindow 类,用于显示一个主窗口的界面。同时,该头文件使用了 Qt 框架提供的信号和槽机制,可以方便地在界面元素之间传递信息。
相关推荐
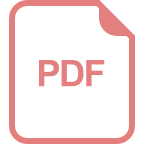












