用遗传算法求解求解二元函数极值问题MATLAB算法
时间: 2023-09-16 20:07:31 浏览: 329
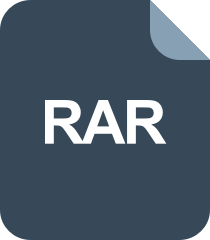
用遗传算法求解函数极值

以下是一个简单的MATLAB代码,用遗传算法来寻找二元函数的最小值:
```matlab
% 定义目标函数
fun = @(x) x(1)^2 + x(2)^2;
% 定义变量的范围
lb = [-10,-10];
ub = [10,10];
% 使用GA算法求解最小值
options = optimoptions('ga','MaxGenerations',100,'PopulationSize',50);
[x,fval] = ga(fun,2,[],[],[],[],lb,ub,[],options);
% 显示最优解和最小值
fprintf('最优解:x1=%f,x2=%f\n',x(1),x(2));
fprintf('最小值:%f\n',fval);
```
在这个例子中,我们定义了一个目标函数 `fun`,它是二元函数 `x1^2 + x2^2`。我们还定义了变量的范围 `lb` 和 `ub`,它们分别是变量 `x1` 和 `x2` 的下限和上限。然后我们使用MATLAB的遗传算法函数 `ga` 来寻找目标函数的最小值。我们还指定了一些选项,如最大代数和种群大小。最后,我们输出最优解和最小值。
阅读全文
相关推荐
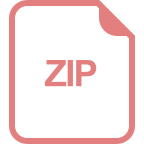
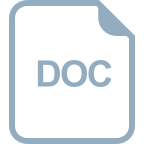


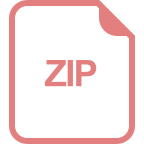
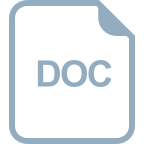



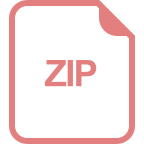
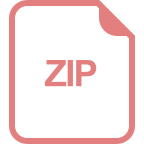
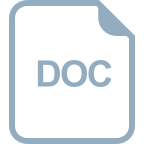
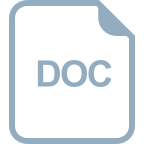



