用C语言生成一个用菜单函数实现的关于二叉树和图的算法管理系统,二叉树和图有一个子菜单,该系统算法包含建立二叉树,输出二叉树,输出二叉树形状,先序遍历,层次遍历,计算节点数,计算叶子节点数,计算树的深度,和用邻接表建立无向图,用邻接矩阵建立无向图,输出图,深度优先遍历,广度优先遍历,普利姆算法求最小生成树,求最短路径的算法
时间: 2023-08-09 16:07:20 浏览: 116
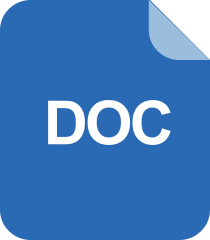
与二叉树有关的算法 c
以下是一个简单的示例代码,包含二叉树和图的菜单函数实现,以及算法管理系统的各个功能:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_NODE 50
// 二叉树节点定义
typedef struct TreeNode {
char data;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 邻接表节点定义
typedef struct GraphNode {
int vertex;
struct GraphNode *next;
} GraphNode;
// 邻接表定义
typedef struct Graph {
int num_vertices;
GraphNode **adj_list;
} Graph;
// 二叉树函数声明
TreeNode *create_tree();
void print_tree(TreeNode *root);
void print_tree_shape(TreeNode *root, int level);
void preorder_traversal(TreeNode *root);
void level_traversal(TreeNode *root);
int count_nodes(TreeNode *root);
int count_leaves(TreeNode *root);
int depth(TreeNode *root);
// 邻接表函数声明
Graph *create_graph();
void add_edge(Graph *graph, int src, int dest);
void print_graph(Graph *graph);
void DFS(Graph *graph, int vertex, int *visited);
void DFS_traversal(Graph *graph, int start_vertex);
void BFS_traversal(Graph *graph, int start_vertex);
void prim_mst(Graph *graph);
void dijkstra_shortest_path(Graph *graph, int start_vertex);
int main() {
int choice, sub_choice;
TreeNode *root = NULL;
Graph *graph = NULL;
do {
printf("\nAlgorithm Management System\n");
printf("1. Binary Tree\n");
printf("2. Graph\n");
printf("3. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
do {
printf("\nBinary Tree Management\n");
printf("1. Create Tree\n");
printf("2. Print Tree\n");
printf("3. Print Tree Shape\n");
printf("4. Preorder Traversal\n");
printf("5. Level Traversal\n");
printf("6. Count Nodes\n");
printf("7. Count Leaves\n");
printf("8. Depth\n");
printf("9. Back to Main Menu\n");
printf("Enter your choice: ");
scanf("%d", &sub_choice);
switch (sub_choice) {
case 1:
root = create_tree();
break;
case 2:
print_tree(root);
break;
case 3:
print_tree_shape(root, 0);
break;
case 4:
preorder_traversal(root);
break;
case 5:
level_traversal(root);
break;
case 6:
printf("Number of Nodes: %d\n", count_nodes(root));
break;
case 7:
printf("Number of Leaves: %d\n", count_leaves(root));
break;
case 8:
printf("Depth of Tree: %d\n", depth(root));
break;
case 9:
break;
default:
printf("Invalid choice!\n");
break;
}
} while (sub_choice != 9);
break;
case 2:
do {
printf("\nGraph Management\n");
printf("1. Create Graph (Adjacency List)\n");
printf("2. Create Graph (Adjacency Matrix)\n");
printf("3. Print Graph\n");
printf("4. Depth First Traversal\n");
printf("5. Breadth First Traversal\n");
printf("6. Prim's Algorithm (Minimum Spanning Tree)\n");
printf("7. Dijkstra's Algorithm (Shortest Path)\n");
printf("8. Back to Main Menu\n");
printf("Enter your choice: ");
scanf("%d", &sub_choice);
switch (sub_choice) {
case 1:
graph = create_graph();
break;
case 2:
printf("This feature is not implemented yet!\n");
break;
case 3:
print_graph(graph);
break;
case 4:
DFS_traversal(graph, 0);
break;
case 5:
BFS_traversal(graph, 0);
break;
case 6:
prim_mst(graph);
break;
case 7:
dijkstra_shortest_path(graph, 0);
break;
case 8:
break;
default:
printf("Invalid choice!\n");
break;
}
} while (sub_choice != 8);
break;
case 3:
printf("Exiting program...\n");
break;
default:
printf("Invalid choice!\n");
break;
}
} while (choice != 3);
return 0;
}
// 二叉树函数实现
TreeNode *create_tree() {
char c;
TreeNode *root;
printf("Enter data (0 for no node): ");
scanf(" %c", &c);
if (c == '0') {
return NULL;
}
root = (TreeNode *) malloc(sizeof(TreeNode));
root->data = c;
root->left = create_tree();
root->right = create_tree();
return root;
}
void print_tree(TreeNode *root) {
if (root != NULL) {
printf("%c ", root->data);
print_tree(root->left);
print_tree(root->right);
}
}
void print_tree_shape(TreeNode *root, int level) {
if (root != NULL) {
print_tree_shape(root->right, level+1);
for (int i = 0; i < level; i++) {
printf(" ");
}
printf("%c\n", root->data);
print_tree_shape(root->left, level+1);
}
}
void preorder_traversal(TreeNode *root) {
if (root != NULL) {
printf("%c ", root->data);
preorder_traversal(root->left);
preorder_traversal(root->right);
}
}
void level_traversal(TreeNode *root) {
TreeNode *queue[MAX_NODE];
int front = 0, rear = 0;
if (root != NULL) {
queue[rear] = root;
rear++;
while (front < rear) {
TreeNode *node = queue[front];
printf("%c ", node->data);
front++;
if (node->left != NULL) {
queue[rear] = node->left;
rear++;
}
if (node->right != NULL) {
queue[rear] = node->right;
rear++;
}
}
}
}
int count_nodes(TreeNode *root) {
if (root == NULL) {
return 0;
} else {
return 1 + count_nodes(root->left) + count_nodes(root->right);
}
}
int count_leaves(TreeNode *root) {
if (root == NULL) {
return 0;
} else if (root->left == NULL && root->right == NULL) {
return 1;
} else {
return count_leaves(root->left) + count_leaves(root->right);
}
}
int depth(TreeNode *root) {
if (root == NULL) {
return 0;
} else {
int left_depth = depth(root->left);
int right_depth = depth(root->right);
if (left_depth > right_depth) {
return left_depth + 1;
} else {
return right_depth + 1;
}
}
}
// 邻接表函数实现
Graph *create_graph() {
int num_vertices, num_edges, src, dest;
Graph *graph = (Graph *) malloc(sizeof(Graph));
printf("Enter number of vertices: ");
scanf("%d", &num_vertices);
graph->num_vertices = num_vertices;
graph->adj_list = (GraphNode **) malloc(num_vertices * sizeof(GraphNode *));
for (int i = 0; i < num_vertices; i++) {
graph->adj_list[i] = NULL;
}
printf("Enter number of edges: ");
scanf("%d", &num_edges);
for (int i = 0; i < num_edges; i++) {
printf("Enter edge (src dest): ");
scanf("%d %d", &src, &dest);
add_edge(graph, src, dest);
add_edge(graph, dest, src);
}
return graph;
}
void add_edge(Graph *graph, int src, int dest) {
GraphNode *new_node = (GraphNode *) malloc(sizeof(GraphNode));
new_node->vertex = dest;
new_node->next = graph->adj_list[src];
graph->adj_list[src] = new_node;
}
void print_graph(Graph *graph) {
for (int i = 0; i < graph->num_vertices; i++) {
GraphNode *current_node = graph->adj_list[i];
printf("Vertex %d: ", i);
while (current_node != NULL) {
printf("%d ", current_node->vertex);
current_node = current_node->next;
}
printf("\n");
}
}
void DFS(Graph *graph, int vertex, int *visited) {
visited[vertex] = 1;
printf("%d ", vertex);
GraphNode *current_node = graph->adj_list[vertex];
while (current_node != NULL) {
int adj_vertex = current_node->vertex;
if (!visited[adj_vertex]) {
DFS(graph, adj_vertex, visited);
}
current_node = current_node->next;
}
}
void DFS_traversal(Graph *graph, int start_vertex) {
int visited[MAX_NODE] = {0};
printf("Depth First Traversal (Starting from vertex %d): ", start_vertex);
DFS(graph, start_vertex, visited);
printf("\n");
}
void BFS_traversal(Graph *graph, int start_vertex) {
int visited[MAX_NODE] = {0};
int queue[MAX_NODE];
int front = 0, rear = 0;
printf("Breadth First Traversal (Starting from vertex %d): ", start_vertex);
visited[start_vertex] = 1;
queue[rear] = start_vertex;
rear++;
while (front < rear) {
int vertex = queue[front];
printf("%d ", vertex);
front++;
GraphNode *current_node = graph->adj_list[vertex];
while (current_node != NULL) {
int adj_vertex = current_node->vertex;
if (!visited[adj_vertex]) {
visited[adj_vertex] = 1;
queue[rear] = adj_vertex;
rear++;
}
current_node = current_node->next;
}
}
printf("\n");
}
void prim_mst(Graph *graph) {
int parent[MAX_NODE];
int key[MAX_NODE];
int visited[MAX_NODE] = {0};
for (int i = 0; i < MAX_NODE; i++) {
key[i] = 9999;
}
key[0] = 0;
parent[0] = -1;
for (int i = 0; i < graph->num_vertices - 1; i++) {
int min_key = 9999, min_vertex = -1;
for (int j = 0; j < graph->num_vertices; j++) {
if (!visited[j] && key[j] < min_key) {
min_key = key[j];
min_vertex = j;
}
}
visited[min_vertex] = 1;
GraphNode *current_node = graph->adj_list[min_vertex];
while (current_node != NULL) {
int adj_vertex = current_node->vertex;
int weight = 1;
if (!visited[adj_vertex] && weight < key[adj_vertex]) {
parent[adj_vertex] = min_vertex;
key[adj_vertex] = weight;
}
current_node = current_node->next;
}
}
printf("Minimum Spanning Tree (Prim's Algorithm): \n");
for (int i = 1; i < graph->num_vertices; i++) {
printf("%d - %d\n", parent[i], i);
}
}
void dijkstra_shortest_path(Graph *graph, int start_vertex) {
int dist[MAX_NODE];
int visited[MAX_NODE] = {0};
for (int i = 0; i < MAX_NODE; i++) {
dist[i] = 9999;
}
dist[start_vertex] = 0;
for (int i = 0; i < graph->num_vertices - 1; i++) {
int min_dist = 9999, min_vertex = -1;
for (int j = 0; j < graph->num_vertices; j++) {
if (!visited[j] && dist[j] < min_dist) {
min_dist = dist[j];
min_vertex = j;
}
}
visited[min_vertex] = 1;
GraphNode *current_node = graph->adj_list[min_vertex];
while (current_node != NULL) {
int adj_vertex = current_node->vertex;
int weight = 1;
if (!visited[adj_vertex] && dist[min_vertex] + weight < dist[adj_vertex]) {
dist[adj_vertex] = dist[min_vertex] + weight;
}
current_node = current_node->next;
}
}
printf("Shortest Path (Dijkstra's Algorithm): \n");
for (int i = 0; i < graph->num_vertices; i++) {
printf("%d - %d: %d\n", start_vertex, i, dist[i]);
}
}
```
阅读全文
相关推荐



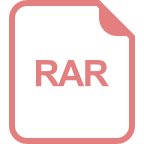
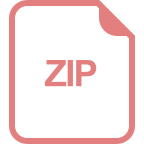
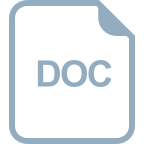
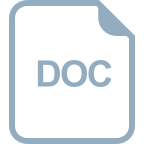
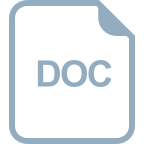
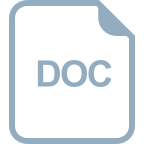
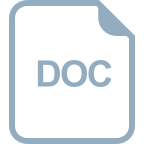
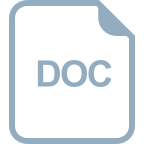
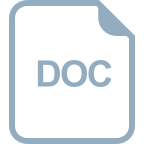
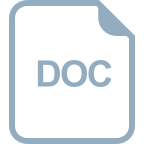
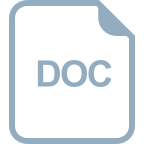
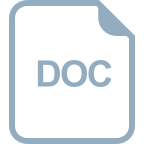
