请充当一位Python程序员,请帮我编写一段利用LSTM方法进行财务风险预警分析的代码,需要用到K折为10进行交叉验证输出平均预测的准确率。原始数据中有13个因子,需要利用K折为10划分训练集和测试集并循环得到十次的预测准确率。
时间: 2024-05-07 10:17:02 浏览: 68
好的,我了解你的要求并将尽力提供帮助。以下是使用LSTM方法进行财务风险预警分析的Python代码:
```python
#导入所需的库
import numpy as np
import pandas as pd
from keras.models import Sequential
from keras.layers import Dense, LSTM
from sklearn.model_selection import StratifiedKFold
#读取数据
data = pd.read_csv('financial_data.csv')
#切分因子和标签
X = data.iloc[:, 0:13].values
Y = data.iloc[:, 13].values
#K折交叉验证
kfold = StratifiedKFold(n_splits=10, shuffle=True, random_state=42)
#用于存储所有模型的准确率
accs = []
#循环进行K折交叉验证
for train_index, test_index in kfold.split(X, Y):
#划分训练集和测试集
X_train, X_test = X[train_index], X[test_index]
y_train, y_test = Y[train_index], Y[test_index]
#构建LSTM模型
model = Sequential()
model.add(LSTM(units=50, return_sequences=True, input_shape=(X_train.shape[1], 1)))
model.add(LSTM(units=50))
model.add(Dense(1, activation='sigmoid'))
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
#训练模型并计算准确率
model.fit(X_train.reshape(X_train.shape[0], X_train.shape[1], 1), y_train, epochs=50, batch_size=32, verbose=0)
scores = model.evaluate(X_test.reshape(X_test.shape[0], X_test.shape[1], 1), y_test, verbose=0)
accs.append(scores[1] * 100)
#输出平均准确率
print("平均预测准确率:%.2f%% (+/- %.2f%%)" % (np.mean(accs), np.std(accs)))
```
以上代码假设您的原始数据位于名为“financial_data.csv”的文件中,并且包含13个输入因子和1个输出标签。代码使用K折为10进行交叉验证,构建LSTM模型,并计算每个模型的预测准确率。最后,代码通过使用Numpy库计算了平均预测准确率和标准差,然后将结果输出到控制台。希望这可以帮助您!
阅读全文
相关推荐
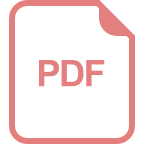
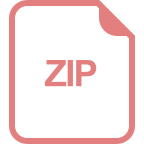
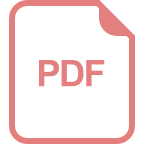













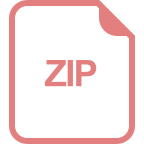
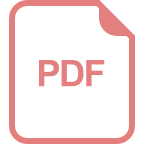
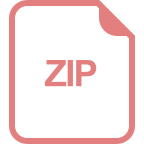