编写java程序统计一篇英语文档中个单词出现的次数,并按单词出现频率由高到低进行输出;
时间: 2023-12-09 08:03:57 浏览: 62
以下是一个简单的 Java 程序,可以实现统计一篇英语文档中单词出现次数并按频率排序的功能:
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.*;
public class WordCount {
public static void main(String[] args) throws FileNotFoundException {
// 读取文件
Scanner scanner = new Scanner(new File("input.txt"));
// 统计每个单词出现的次数
Map<String, Integer> wordCountMap = new HashMap<>();
while (scanner.hasNext()) {
String word = scanner.next().toLowerCase();
if (wordCountMap.containsKey(word)) {
wordCountMap.put(word, wordCountMap.get(word) + 1);
} else {
wordCountMap.put(word, 1);
}
}
// 将结果按照出现次数排序
List<Map.Entry<String, Integer>> sortedWordCountList = new ArrayList<>(wordCountMap.entrySet());
sortedWordCountList.sort((o1, o2) -> o2.getValue() - o1.getValue());
// 输出结果
for (Map.Entry<String, Integer> entry : sortedWordCountList) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
```
其中,`input.txt` 是输入文件的文件名,程序通过 `Scanner` 读取输入文件中的单词,并使用 `HashMap` 统计每个单词出现的次数。然后,将统计结果按照出现次数排序,并输出结果。需要注意的是,为了统计的准确性,程序在读取每个单词时将其转换成小写字母。
相关推荐
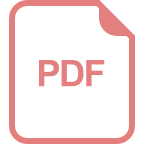
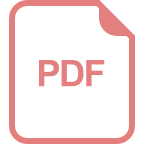
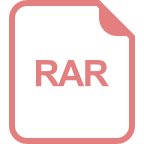














