使用c 语言编写spi 时序
时间: 2024-03-07 21:46:52 浏览: 53
SPI(Serial Peripheral Interface)是一种同步串行通信协议,常用于与外围设备进行通信。下面是使用 C 语言编写 SPI 时序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <linux/spi/spidev.h>
int main(int argc, char *argv[])
{
int fd;
unsigned char mode = SPI_MODE_0; // SPI 模式
unsigned char bits_per_word = 8; // 每个字节的位数
unsigned int speed_hz = 1000000; // 时钟频率
// 打开 SPI 设备
fd = open("/dev/spidev0.0", O_RDWR);
if (fd < 0) {
perror("Failed to open SPI device");
exit(1);
}
// 设置 SPI 模式
if (ioctl(fd, SPI_IOC_WR_MODE, &mode) < 0) {
perror("Failed to set SPI mode");
exit(1);
}
// 设置每个字节的位数
if (ioctl(fd, SPI_IOC_WR_BITS_PER_WORD, &bits_per_word) < 0) {
perror("Failed to set bits per word");
exit(1);
}
// 设置时钟频率
if (ioctl(fd, SPI_IOC_WR_MAX_SPEED_HZ, &speed_hz) < 0) {
perror("Failed to set clock speed");
exit(1);
}
// 发送数据
unsigned char send_buf[2] = {0x01, 0x23};
struct spi_ioc_transfer transfer = {
.tx_buf = (unsigned long)send_buf,
.rx_buf = 0,
.len = sizeof(send_buf),
.delay_usecs = 0,
.speed_hz = speed_hz,
.bits_per_word = bits_per_word,
.cs_change = 0,
};
if (ioctl(fd, SPI_IOC_MESSAGE(1), &transfer) < 0) {
perror("Failed to send SPI message");
exit(1);
}
// 关闭 SPI 设备
close(fd);
return 0;
}
```
该示例代码中使用了 Linux SPI 设备驱动程序,需要在编译时链接 `libspi-dev` 库。编译命令如下:
```
gcc -o spi_test spi_test.c -lspi-dev
```
其中,`spi_test.c` 是示例代码的文件名。执行 `./spi_test` 命令即可运行该程序。
阅读全文
相关推荐

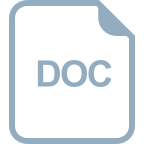
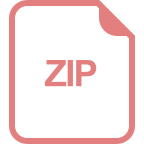
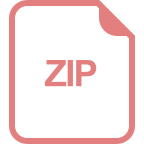
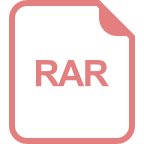
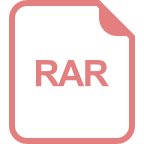
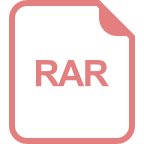
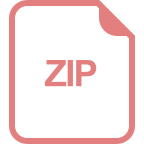
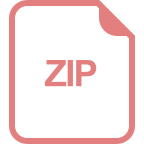
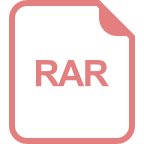
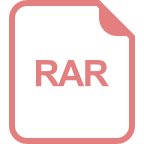
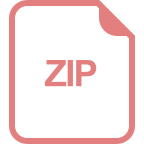
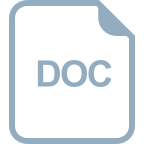
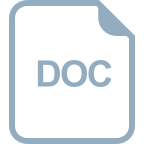
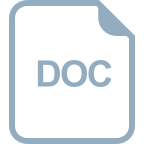
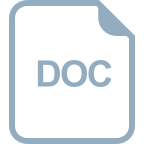