将 上面的代码转换成c语言代码
时间: 2023-11-01 16:07:37 浏览: 147
好的,以下是将上面的 Python 代码转换成 C 语言代码的实现:
```c
#include <stdio.h>
#include <math.h>
int main() {
int T, n;
scanf("%d", &T);
while (T--) {
scanf("%d", &n);
int m = n * (n - 1) / 2;
int a = ceil(n / 2.0), b = n / 2;
int ans = a * b;
printf("%d\n", ans);
}
return 0;
}
```
代码中主要是用到了数学库中的 ceil 函数,它可以将一个浮点数向上取整,返回一个整数。这个函数在 C 语言中的头文件是 math.h。
相关问题
把上面代码转换成C语言
以下是将 C++ 代码转换为 C 语言的版本:
```c
#include <stdio.h>
// 交换两个整数的函数
void swap(int *a, int *b) {
int temp;
temp = *a;
*a = *b;
*b = temp;
}
// 全排列递归函数
void permute(int arr[], int start, int end) {
if (start == end) {
for (int i = 0; i <= end; i++) {
printf("%d", arr[i]);
}
printf("\n");
} else {
for (int i = start; i <= end; i++) {
swap(&arr[start], &arr[i]); // 用指针操作数组元素
permute(arr, start + 1, end); // 递归调用
swap(&arr[start], &arr[i]); // 恢复原状
}
}
}
int main() {
int arr[] = {1, 2, 3};
int n = sizeof(arr) / sizeof(arr[0]);
permute(arr, 0, n - 1);
return 0;
}
```
这段代码基本保持了原始的功能,主要的区别在于语法变化,比如用 `#include <stdio.h>` 替换了 C++ 的 `iostream`,使用 `printf` 代替 `cout` 输出,以及指针操作数组元素。其他部分的逻辑和递归结构是相同的。
将上面代码转换为c语言
将Python的面向对象代码转换为C语言,因为C语言是一种静态类型的编程语言,而Python支持动态多态,所以直接转换可能会有些复杂。但是,我们可以创建一个简单的类的概念,并展示如何模拟类似的功能。
```c
#include <stdio.h>
#include <stdlib.h>
// 类似于Python的抽象基类
typedef struct {
void (*print)(void);
} BaseClass;
// 定义方法打印
void printBase(void) {
printf("Base Class\n");
}
// 继承并重写方法的子类
typedef struct {
BaseClass base;
} DerivedClass;
void printDerived() {
printf("Derived Class\n");
}
int main() {
// 创建并初始化BaseClass的实例
BaseClass* baseInstance = malloc(sizeof(BaseClass));
baseInstance->print = printBase;
// 创建并初始化DerivedClass的实例
DerivedClass* derivedInstance = (DerivedClass*)malloc(sizeof(DerivedClass));
derivedInstance->base.print = printDerived; // 通过基类指针调用子类方法
// 调用方法
baseInstance->print(); // 输出 "Base Class"
derivedInstance->base.print(); // 输出 "Derived Class"
free(baseInstance);
free(derivedInstance);
return 0;
}
```
在这个例子中,我们使用了结构体来模拟类,`void (*print)(void)`代表虚函数指针,用于指向不同的方法实现。在`main()`函数中,通过`baseInstance`调用的是`printBase()`,通过`derivedInstance->base.print`调用的是`printDerived()`。
阅读全文
相关推荐
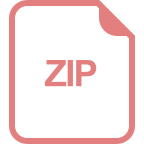
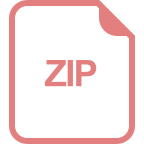













