请编写一个Dyna-Q算法的代码
时间: 2024-03-27 18:39:27 浏览: 53
好的,以下是一个简单的Dyna-Q算法的Python代码实现:
```python
import numpy as np
# 定义环境
n_states = 6
n_actions = 2
T = np.zeros((n_states, n_actions, n_states))
R = np.zeros((n_states, n_actions, n_states))
T[0, 0, :] = [0.7, 0.3, 0, 0, 0, 0]
T[0, 1, :] = [0, 0.3, 0.7, 0, 0, 0]
T[1, :, :] = [0.3, 0.7, 0, 0, 0, 0]
T[2, :, :] = [0, 0, 0, 0.8, 0, 0.2]
T[3, :, :] = [0, 0, 0, 0.4, 0.6, 0]
T[4, :, :] = [0, 0, 0, 0, 0.5, 0.5]
T[5, :, :] = [0, 0, 0, 0, 0, 1]
R[0, 0, :] = [-0.1, -0.1, 0, 0, 0, 1]
R[0, 1, :] = [0, -0.1, -0.1, 0, 0, 1]
R[1, :, :] = [-0.1, 0, 0, 0, 0, 1]
R[2, :, :] = [0, 0, 0, -0.1, 0, 1]
R[3, :, :] = [0, 0, 0, -0.1, -0.1, 1]
R[4, :, :] = [0, 0, 0, 0, -0.1, 1]
R[5, :, :] = [0, 0, 0, 0, 0, 0]
# 定义参数
alpha = 0.5
gamma = 0.9
epsilon = 0.1
n_planning = 5
n_episodes = 1000
# 初始化Q值和模型
Q = np.zeros((n_states, n_actions))
model_T = np.zeros((n_states, n_actions, n_states))
model_R = np.zeros((n_states, n_actions, n_states))
# Dyna-Q算法主循环
for i in range(n_episodes):
# 初始化状态
state = np.random.randint(n_states)
# 每个episode内进行多次更新
for j in range(100):
# 按照epsilon-greedy策略选择动作
if np.random.uniform() < epsilon:
action = np.random.randint(n_actions)
else:
action = np.argmax(Q[state, :])
# 执行动作并观察奖励和新状态
new_state = np.random.choice(n_states, p=T[state, action, :])
reward = R[state, action, new_state]
# 更新Q值
Q[state, action] = Q[state, action] + alpha * (reward + gamma * np.max(Q[new_state, :]) - Q[state, action])
# 更新模型
model_T[state, action, new_state] = model_T[state, action, new_state] + 1
model_R[state, action, new_state] = reward
# planning
for k in range(n_planning):
# 从模型中采样一个先前经历过的状态和动作
prev_state = np.random.randint(n_states)
prev_action = np.random.randint(n_actions)
# 通过模型预测下一个状态和奖励
next_state = np.argmax(model_T[prev_state, prev_action, :])
reward = model_R[prev_state, prev_action, next_state]
# 更新Q值
Q[prev_state, prev_action] = Q[prev_state, prev_action] + alpha * (reward + gamma * np.max(Q[next_state, :]) - Q[prev_state, prev_action])
# 更新状态
state = new_state
```
以上代码实现了一个简单的Dyna-Q算法,用于解决一个简单的MDP问题。算法的主要思路是利用模型(T和R)来生成先前经历的状态和动作的概率分布,并对这些状态和动作进行多次更新,以提高算法的性能。在代码实现中,我们使用了一个`model_T`和`model_R`来存储从环境中观测到的先前经历的状态和动作,每次planning时从中采样。
阅读全文
相关推荐
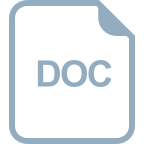
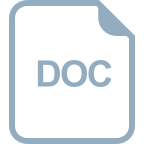
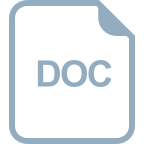


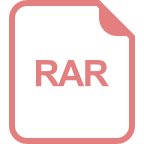






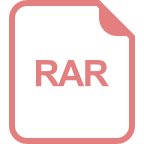
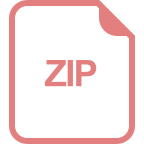
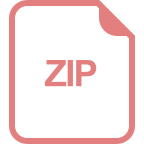
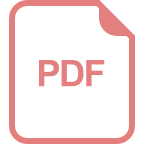
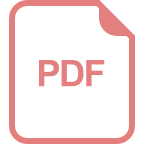
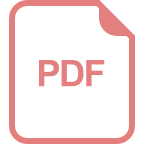
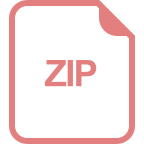