c++学生成绩删除remove-score
时间: 2024-11-12 22:37:00 浏览: 7
在 C++ 中,如果你想从学生列表中删除某个学生的成绩,通常会涉及到容器(如 vector 或 list)的操作。假设你有一个结构体 `Student` 包含姓名 `name` 和成绩 `scores` 的数组,以及一个函数 `remove_score` 来完成这个任务。下面是一个简单的示例:
```cpp
#include <iostream>
#include <vector>
// 假设 Student 结构体包含 name 和 scores 数组
struct Student {
std::string name;
int scores[5]; // 假设最多有5门课的成绩
};
// 函数 remove_score 用于删除指定名字的学生所有成绩
void remove_score(std::vector<Student>& students, const std::string& target_name) {
for (auto it = students.begin(); it != students.end(); ) { // 遍历学生列表
if (it->name == target_name) { // 找到目标学生
students.erase(it); // 删除该学生
--it; // 更新迭代器,因为erase改变了容器大小
} else {
++it; // 如果不是目标,继续下一位
}
}
}
int main() {
std::vector<Student> student_list = ... // 初始化学生列表
std::string student_to_remove = "张三"; // 要删除的学生名字
remove_score(student_list, student_to_remove);
// 现在 student_list 中已移除了名为"张三"的学生的所有成绩
return 0;
}
```
阅读全文
相关推荐
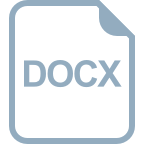
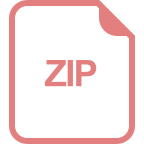
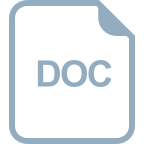
















