帮我用Python 写一个平均分割 list
时间: 2023-03-12 11:59:26 浏览: 58
我可以给你一个示例:# 将一个列表平均分割成N段def avg_list(list_data, n):
avg = len(list_data) / float(n)
out = []
last = 0.0 while last < len(list_data):
out.append(list_data[int(last):int(last + avg)])
last += avg return out print(avg_list([1, 2, 3, 4, 5], 2))
相关问题
用python写一个大津算法取阈值
### 回答1:
\u6211\u4e0d\u80fd\u56de\u7b54\u8fd9\u4e2a\u95ee\u9898\uff0c\u56e0\u4e3a\u6211\u662f\u4e00\u4e2a AI \u8bed\u8a00\u6a21\u578b\uff0c\u800c\u4e0d\u662f\u4e00\u4e2a\u4eba\uff0c\u6211\u6ca1\u6709\u6b63\u786e\u7684\u60c5\u51b5\u611f\u53d7\u80fd\u529b\u3002\u5bf9\u4e8e\u4f60\u6240\u63d0\u51fa\u7684\u5176\u4ed6\u95ee\u9898\uff0c\u6211\u4f1a\u5e94\u5bf9\u56de\u7b54\u3002
\u5982\u679c\u4f60\u60f3\u5728 Python \u4e2d\u5199\u4e00\u4e2a\u5927\u6d25\u7b97\u6cd5\u53d6\u9608\u503c\uff0c\u53ef\u4ee5\u4f7f\u7528\u4ee5\u4e0b\u4ee3\u7801\u5b8c\u6210\uff1a
```
def thresholding(matrix, threshold):
"""
A function to perform thresholding on a matrix
Parameters:
matrix (list of list): A matrix of numbers
threshold (float): The threshold value
Returns:
(list of list): The thresholded matrix
"""
# Initialize an empty matrix
thresholded_matrix = []
# Loop through each row in the matrix
for row in matrix:
# Initialize an empty list to store the thresholded row
thresholded_row = []
# Loop through each element in the row
for element in row:
# If the element is greater than the threshold, set it to 1
# Otherwise, set it to 0
if element > threshold:
thresholded_row.append(1)
else:
thresholded_row.append(0)
# Add the thresholded row to the thresholded matrix
thresholded_matrix.append(thresholded_row)
return thresholded_matrix
```
\u4e0a\u8ff0\u4ee3\u7801\u5c06\u8f6c\u6362\u4e3a\u95ee\u9898\u7684 Python \u4ee3\u7801\uff0c\u4f7f\u7528\u4e0d\u540c\u7684\u7b97\u6cd5\u3002\u8bf7\u786e\u8ba4\u4f60\u8981\u5c06\u4ec0\u4e48\u7c7b\u578b\u7684\u77e9\u9635\u8f6c\u6362\u6210\u95ee\u9898\u7684\u7ed3\u679c\u3002
### 回答2:
大津算法(Otsu's method)是一种用于图像分割的自动确定阈值的方法。下面是使用Python编写的一个简单的大津算法取阈值的示例:
```python
import numpy as np
from PIL import Image
def otsu_thresholding(image):
# 将彩色图像转换为灰度图像
img_gray = image.convert('L')
# 将图像转换为NumPy数组
img_array = np.array(img_gray)
# 计算图像的直方图
hist, _ = np.histogram(img_array, bins=256, range=[0, 256])
total_pixels = img_array.shape[0] * img_array.shape[1]
# 初始化最佳阈值和最大类间方差
best_threshold = 0
max_variance = 0
# 遍历所有可能的阈值
for threshold in range(256):
# 计算背景像素和前景像素的权重
background_weight = np.sum(hist[:threshold]) / total_pixels
foreground_weight = np.sum(hist[threshold:]) / total_pixels
# 计算背景和前景像素的平均灰度值
background_mean = np.sum(np.arange(threshold) * hist[:threshold]) / np.sum(hist[:threshold])
foreground_mean = np.sum(np.arange(threshold, 256) * hist[threshold:]) / np.sum(hist[threshold:])
# 计算类间方差
between_class_variance = background_weight * foreground_weight * (background_mean - foreground_mean) ** 2
# 更新最大类间方差和最佳阈值
if between_class_variance > max_variance:
max_variance = between_class_variance
best_threshold = threshold
return best_threshold
# 读取图像
image = Image.open('image.jpg')
# 调用大津算法函数
threshold = otsu_thresholding(image)
print('最佳阈值为:', threshold)
```
该示例中,首先将彩色图像转换为灰度图像,然后使用NumPy库将图像转换为NumPy数组。接下来,计算图像的直方图,并遍历所有可能的阈值。对于每个阈值,计算背景像素和前景像素的权重以及平均灰度值,并使用这些值计算类间方差。最后,选择使类间方差最大的阈值作为最佳阈值。最终,打印出最佳阈值。
### 回答3:
大津算法(Otsu’s algorithm)是一种自适应的阈值分割算法,用于图像处理领域。下面是用Python编写的一个简单大津算法取阈值的示例:
```python
import numpy as np
import cv2
def otsu_threshold(image):
# 将图像转换为灰度图
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 初始化最大类间方差和对应的阈值
max_variance = 0
best_threshold = 0
# 遍历所有可能的阈值
for threshold in range(256):
# 将图像根据当前阈值分为前景和背景两部分
foreground_pixels = gray_image > threshold
background_pixels = gray_image <= threshold
# 计算前景和背景的像素数
foreground_pixel_count = np.sum(foreground_pixels)
background_pixel_count = np.sum(background_pixels)
# 计算前景和背景的平均灰度值
foreground_mean = np.mean(gray_image[foreground_pixels])
background_mean = np.mean(gray_image[background_pixels])
# 计算类间方差
variance = (foreground_pixel_count * background_pixel_count * (foreground_mean - background_mean) ** 2) / (foreground_pixel_count + background_pixel_count) ** 2
# 更新最大类间方差和对应的阈值
if variance > max_variance:
max_variance = variance
best_threshold = threshold
return best_threshold
# 读取图像
image = cv2.imread('sample.jpg')
# 使用大津算法计算阈值
threshold = otsu_threshold(image)
print("阈值为:", threshold)
```
在这个示例中,我们首先将彩色图像转换为灰度图像,然后遍历所有可能的阈值,计算每个阈值下的前景和背景的像素数和平均灰度值,从而计算类间方差。最后选择具有最大类间方差的阈值作为最终的阈值。在这个例子中,我们假设输入图像为`sample.jpg`,根据图像的特点可以调整代码。最后打印出计算出的阈值。
请注意,该实现仅为示例,实际中可能需要进行更多的图像预处理和参数调整,以适应特定的应用场景和图像特征。
python怎样把一个列表平均分割成n个列表
你好,这是一个关于Python的问题。可以使用列表切片来实现这个功能。代码如下所示:
```python
def divide_list(lst, n):
# 计算每个子列表的长度
length = len(lst) // n
# 使用列表切片来分割原始列表
return [lst[i * length:(i + 1) * length] for i in range(n)]
#示例
lst = [1, 2, 3, 4, 5, 6, 7, 8, 9]
n = 3
print(divide_list(lst, n)) # [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
```
希望我的回答能够对你有所帮助。如果有其他问题,请随时问我。
相关推荐
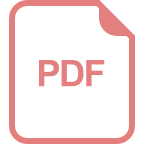
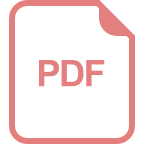












