vue3语法糖prop传参
时间: 2023-09-11 19:04:58 浏览: 46
Vue 3 中使用 props 传递参数的语法糖如下:
1. 在组件中定义 props 属性:
```
// MyComponent.vue
<template>
<div>{{ message }}</div>
</template>
<script>
export default {
props: {
message: String
}
}
</script>
```
2. 在父组件中使用 props:
```
// ParentComponent.vue
<template>
<my-component :message="helloWorld" />
</template>
<script>
import MyComponent from './MyComponent.vue'
export default {
components: {
MyComponent
},
data() {
return {
helloWorld: 'Hello, World!'
}
}
}
</script>
```
在父组件中使用 `:` 将 `helloWorld` 传递给 `MyComponent` 组件的 `message` 属性。注意,这里的 `helloWorld` 是一个字符串类型的数据,因为在 `MyComponent` 中定义了 `message` 的类型为 `String`。
相关问题
vue3setup语法糖prop父传子
在 Vue3 的 setup 函数中,如果需要进行父组件向子组件传值,可以使用 prop 语法糖。
在子组件中,可以使用 defineProps 函数来定义需要接收的 props,如下所示:
```
import { defineProps } from 'vue'
export default {
props: {
msg: String
},
setup(props) {
// 使用 props.msg 进行操作
}
}
```
在父组件中,可以直接将需要传递的值作为子组件的属性进行传递,如下所示:
```
<template>
<ChildComponent msg="Hello World" />
</template>
<script>
import ChildComponent from './ChildComponent.vue'
export default {
components: {
ChildComponent
}
}
</script>
```
这样,在子组件中,就可以通过 props.msg 来获取父组件传递的值了。如果需要进行类型检查,可以使用 defineProps 函数来定义 props 的类型和默认值。例如:
```
import { defineProps } from 'vue'
export default {
props: {
msg: String
},
setup(props) {
// 使用 props.msg 进行操作
}
}
// 使用 defineProps 进行类型检查
const props = defineProps({
msg: {
type: String,
default: 'Hello World'
}
});
```
webstorm配置vue3语法糖
要在WebStorm中配置Vue 3语法糖,你可以按照以下步骤进行操作:
1. 确保你的WebStorm版本是最新的,以便支持Vue 3的新特性。
2. 打开WebStorm的设置(Settings)。你可以通过菜单栏的"File" -> "Settings"或使用快捷键Ctrl + Alt + S来访问设置。
3. 在设置窗口中,选择"Editor" -> "Live Templates"。
4. 在左侧的面板中,选择适当的语言,如HTML或Vue。
5. 点击右侧的"+"按钮或使用快捷键Alt + Insert来添加新的Live Template。
6. 输入你想要的快捷键缩写,例如`vfor`。
7. 在"Template text"字段中,输入Vue 3语法糖的代码片段。例如,对于v-for指令,你可以输入`v-for="item in items"`。
8. 配置其他选项,如"Applicable in"(适用于)和"By default"(默认情况下),根据需要进行调整。
9. 点击"OK"按钮保存并应用设置。
现在,当你在Vue文件中输入你配置的快捷键缩写并按下Tab键时,WebStorm将会自动插入对应的Vue 3语法糖代码片段。
通过配置Vue 3语法糖,你可以更快速地编写Vue 3代码,并提高开发效率。同时,你也可以根据需要添加其他Vue 3语法糖配置,以满足你的开发需求。
相关推荐
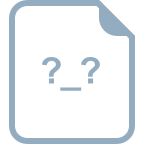












