ts.functools_lru_cache
时间: 2024-12-20 20:18:38 浏览: 15
`ts.functools_lru_cache` 是 Python 标准库 `functools` 模块中的一个装饰器,全称为 Least Recently Used (LRU) 缓存。它用于缓存函数的结果,以便当函数被多次调用时,如果某个函数的输入值已经计算过,就可以直接从缓存中返回结果,而无需再次计算,提高了性能。
装饰器 `@lru_cache(maxsize=None)` 可以应用到任何函数上,其中 `maxsize` 参数是可选的,默认值为 None,表示无限缓存大小。如果设置了一个具体的整数,那么当缓存达到这个大小时,最久未使用的项会被自动移除,以保持内存的高效利用。
使用这个装饰器的例子如下:
```python
from functools import lru_cache
@lru_cache(maxsize=10)
def expensive_function(n):
# 这是一个昂贵的操作
return n * n
# 输出会快速返回,因为之前的结果会被缓存
print(expensive_function(5)) # 输出: 25
```
相关问题
@functools.lru_cache
`functools.lru_cache` 是 Python 标准库中的一个装饰器函数,它用于实现最近最少使用缓存(Least Recently Used Cache)。当函数被装饰时,它会缓存函数的结果,以便在相同的参数被传递给函数时,可以直接返回缓存的结果,而不需要重新计算。
LRU 缓存是一种常见的缓存策略,它会保留最近被访问的一部分缓存项,而淘汰掉最久未被访问的缓存项。`functools.lru_cache` 提供了一个方便的方式来使用 LRU 缓存策略,它接受一个可选的 `maxsize` 参数,用于指定缓存的最大大小。
要使用 `functools.lru_cache`,只需将其作为装饰器应用于你想要缓存的函数即可。下面是一个简单的示例:
```python
from functools import lru_cache
@lru_cache(maxsize=128)
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
fibonacci(10) # 第一次调用会计算并缓存结果
fibonacci(10) # 直接返回缓存的结果,无需重新计算
```
在上面的示例中,`fibonacci` 函数使用了 `functools.lru_cache` 装饰器,并设置了最大缓存大小为 128。当第一次调用 `fibonacci(10)` 时,会计算并缓存结果。第二次调用时,直接从缓存中返回结果,无需重新计算。
这是 `functools.lru_cache` 的基本用法,它可以帮助提高函数的性能,特别是对于那些计算开销较大的函数。
ImportError: No module named functools_lru_cache
This error occurs when the Python code is trying to import and use the module "functools_lru_cache", but the module is not installed or cannot be found.
To resolve this error, you can try the following steps:
1. Check if the module is installed: Run the command `pip freeze` in the terminal/command prompt to see if the module is listed. If not, install it using `pip install functools_lru_cache`.
2. Check if the module is spelled correctly: Ensure that the module name is spelled correctly in the import statement.
3. Check the Python version: The module "functools_lru_cache" is available from Python 3.2 onwards. If you're using an older version of Python, upgrade it to a newer version.
4. Check the environment: Ensure that you're running your Python code in the correct environment. If you're using virtual environments, activate the correct environment before running the code.
5. Check the file path: Make sure that the file containing the module is in the correct file path and can be accessed by the Python interpreter.
If none of these solutions work, you may need to provide more information about your specific situation and code for further assistance.
阅读全文
相关推荐


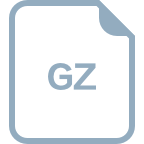













