回答其中的问题 并生成完整代码
时间: 2024-12-24 07:23:57 浏览: 3
为了适应您提供的 JSON 文件格式,并确保代码能够正确处理这些数据,我将对原始代码进行必要的修改。以下是修改后的完整代码:
```python
import json
import datetime
import os
import gr # 假设这是 Gradio 库
def d_show_absent_personInfo(absentInfo, j, yd, qql):
global all_records # 使用 global 关键字来访问外部定义的 all_records 列表
all_records_list = [] # 使用列表来存储每次生成的 all_records,以便后续计算交集
json_file_counter = 0 # 用于跟踪已生成的 JSON 文件数量
def write_all_records_to_file(records, filename):
"""将记录写入 JSON 文件"""
with open(filename, 'w', encoding='utf-8') as file:
json.dump(records, file, indent=4, ensure_ascii=False)
def calculate_intersection(records_list):
"""计算多个字典列表的交集"""
if not records_list:
return []
# 假设每个记录都是一个字典,且字典的键是日期,值是另一个字典
# 我们只对日期对应的值(即另一个字典)计算交集
intersection = records_list[0]
for record in records_list[1:]:
# 对每个日期的值(字典)求交集
new_intersection = []
for item in intersection:
date = next(iter(item.keys()))
data = item[date]
common_items = [r for r in record if date in r and r[date] == data]
if common_items:
new_intersection.append(item)
intersection = new_intersection
return intersection
# 初始化或重置 all_records 列表(但这里我们实际上用不到它,因为我们会直接操作 all_records_list)
# all_records = [] # 这行可以删除或注释掉
sd = yd * qql
if absentInfo is None:
return
absentNum = len(absentInfo)
if absentNum > 0:
with gr.Row():
gr.Markdown(f"# 本堂课有 {absentNum} 位缺勤人员,其详细信息为:")
else:
with gr.Row():
gr.Markdown("# 本堂课没有缺勤人员。")
return
for index, content in enumerate(absentInfo):
try:
# 读取 JSON 文件
with open(content['cfgfilepath'], 'r') as file:
record = json.load(file)
record_time = datetime.datetime.now().strftime('%Y-%m-%d')
data = {
"Class A": f"今天应到 {yd} 人实到 {sd} 人",
"Class B": f"今天应到 {yd} 人实到 {sd} 人"
}
for item in record:
item[record_time] = data
all_records_list.append(record) # 将记录添加到列表中,而不是全局变量
# (其他处理逻辑,如检查字段等,可以放在这里)
except FileNotFoundError:
print("文件未找到,请检查文件路径。", content['cfgfilepath'])
except json.JSONDecodeError:
print("JSON 格式错误,请检查文件内容。", content['cfgfilepath'])
# 循环结束后,增加 json_file_counter 的值
json_file_counter += 1
print(j, json_file_counter)
# 构造文件名并写入文件
new_file_path = f'/home2/anz/Anz1/ccc/re{j}.json'
write_all_records_to_file(all_records_list[-1], new_file_path) # 这里只写入了最后一个记录,但我们需要处理交集
# 检查是否需要计算交集
if json_file_counter % 3 == 0:
# 获取最后三个文件的路径(假设它们是按顺序生成的)
intersection_files = [
f'/home2/anz/Anz1/ccc/re{j-2}.json',
f'/home2/anz/Anz1/ccc/re{j-1}.json',
new_file_path
]
# 读取这三个文件的内容
intersection_records = []
for filepath in intersection_files:
if os.path.exists(filepath):
with open(filepath, 'r', encoding='utf-8') as file:
intersection_records.extend(json.load(file))
else:
print(f"文件 {filepath} 不存在,无法计算交集。")
intersection_records = []
break # 如果有一个文件不存在,就停止计算交集
# 计算交集
if intersection_records:
intersection_result = calculate_intersection(intersection_records)
# 构造交集文件的路径
intersection_file_path = f'/home2/anz/Anz1/ccd/intersection_{j}.json'
# 将交集结果写入文件
write_all_records_to_file(intersection_result, intersection_file_path)
print(f"交集文件已生成:{intersection_file_path}")
```
### 主要修改点:
1. **JSON 文件结构适配**:在读取 JSON 文件时,假设每个文件中的记录是一个列表,每个列表项是一个字典。
2. **交集计算**:`calculate_intersection` 函数被修改为处理包含多个字典的列表,并计算这些字典在特定日期下的交集。
3. **文件读取和写入**:确保文件读取和写入操作能够正确处理 JSON 数据。
希望这些修改能够满足您的需求。如果有任何进一步的问题或需要调整的地方,请随时告诉我。
阅读全文
相关推荐
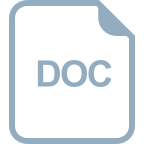
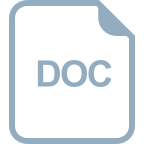
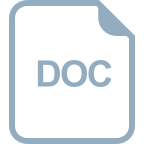















