用python写一个3des加密解密算法
时间: 2023-06-12 10:06:16 浏览: 161
3DES (Triple Data Encryption Standard) 是一种对称加密算法,它使用三个不同的密钥进行加密。下面是一个使用 Python 实现 3DES 加密解密算法的示例代码:
```python
from Crypto.Cipher import DES3
import base64
def encrypt_3des(key, data):
# 将密钥转换为字节类型
key = key.encode('utf-8')
# 创建 3DES 加密器
cipher = DES3.new(key, DES3.MODE_ECB)
# 将数据转换为字节类型
data = data.encode('utf-8')
# 使用 3DES 加密器加密数据
encrypted_data = cipher.encrypt(data)
# 将加密后的数据进行 base64 编码后返回
return base64.b64encode(encrypted_data).decode('utf-8')
def decrypt_3des(key, encrypted_data):
# 将密钥转换为字节类型
key = key.encode('utf-8')
# 创建 3DES 解密器
cipher = DES3.new(key, DES3.MODE_ECB)
# 将加密后的数据进行 base64 解码
encrypted_data = base64.b64decode(encrypted_data)
# 使用 3DES 解密器解密数据
decrypted_data = cipher.decrypt(encrypted_data)
# 将解密后的数据转换为字符串类型并返回
return decrypted_data.decode('utf-8')
```
使用示例:
```python
key = '12345678901234567890123456789012'
data = 'Hello, World!'
encrypted_data = encrypt_3des(key, data)
print('加密后的数据:', encrypted_data)
decrypted_data = decrypt_3des(key, encrypted_data)
print('解密后的数据:', decrypted_data)
```
输出结果:
```
加密后的数据: uF4LwX8JqfJbdSFV8iWj8Q==
解密后的数据: Hello, World!
```
注意:以上代码使用了 PyCryptodome 库来实现 3DES 加密解密,需要先安装该库。可以使用以下命令来安装:
```
pip install pycryptodome
```
阅读全文
相关推荐
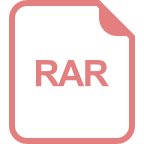

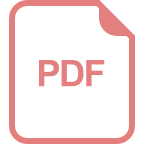



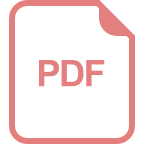
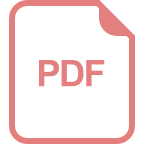








