利用线性表实现通讯录管理
时间: 2023-05-24 09:03:21 浏览: 54
A:通讯录是一种典型的线性表应用场景。我们可以利用线性表(如数组、链表)实现通讯录的管理。
以数组为例,可以先定义一个结构体表示联系人信息:
```
struct Contact {
char name[20]; // 姓名
char tel[15]; // 电话号码
char email[30]; // 电子邮件
};
```
然后创建一个数组来存储联系人信息:
```
#define MAX_CONTACTS 100 // 最多存储100个联系人
Contact contacts[MAX_CONTACTS]; // 存储联系人信息的数组
int numContacts = 0; // 当前联系人数量
```
可以实现以下基本的功能:
1. 添加联系人:
```
bool addContact(const char* name, const char* tel, const char* email) {
if (numContacts >= MAX_CONTACTS) {
return false; // 联系人数量已达上限,添加失败
}
Contact newContact;
strcpy(newContact.name, name);
strcpy(newContact.tel, tel);
strcpy(newContact.email, email);
contacts[numContacts] = newContact;
numContacts++;
return true; // 添加成功
}
```
2. 删除联系人:
```
bool removeContact(int index) {
if (index < 0 || index >= numContacts) {
return false; // 删除的索引不合法,删除失败
}
for (int i = index; i < numContacts - 1; i++) {
contacts[i] = contacts[i + 1]; // 将后面的联系人向前移动
}
numContacts--;
return true; // 删除成功
}
```
3. 搜索联系人:
```
void searchContact(const char* name) {
bool found = false;
for (int i = 0; i < numContacts; i++) {
if (strcmp(contacts[i].name, name) == 0) {
printf("姓名:%s,电话:%s,电子邮件:%s\n",
contacts[i].name, contacts[i].tel, contacts[i].email);
found = true;
}
}
if (!found) {
printf("未找到姓名为%s的联系人\n", name);
}
}
```
4. 修改联系人:
```
bool modifyContact(int index, const char* tel, const char* email) {
if (index < 0 || index >= numContacts) {
return false; // 修改的索引不合法,修改失败
}
strcpy(contacts[index].tel, tel);
strcpy(contacts[index].email, email);
return true; // 修改成功
}
```
以上是利用数组实现通讯录管理的一个示例,当然也可以使用链表等其他数据结构来实现。
相关推荐
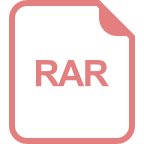
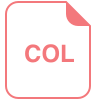
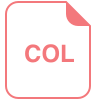
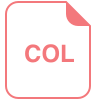
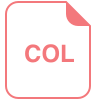
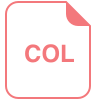









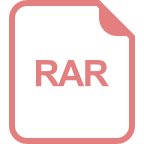