) 根据输入的灰度图像,分别计算图像的均值、方差等统计特征,并计算图像的直方图特征并以图形方式显示图像的直方图(用C或C++语言实现)。
时间: 2024-02-12 15:07:57 浏览: 100
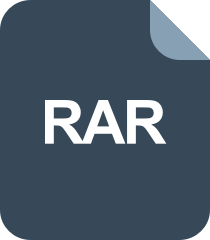
灰度图像直方图计算

好的,我可以为您解答。针对您的问题,以下是一个简单的 C++ 代码示例,可以实现计算图像的均值、方差以及直方图特征。需要注意的是,该示例仅支持灰度图像,对于彩色图像需要进行相应的处理。
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main(int argc, char** argv)
{
// 加载图像
Mat img = imread("test.png", IMREAD_GRAYSCALE);
// 计算图像均值与方差
Scalar mean, stddev;
meanStdDev(img, mean, stddev);
cout << "Mean: " << mean[0] << endl;
cout << "Stddev: " << stddev[0] << endl;
// 计算图像直方图
const int histSize[] = { 256 };
const float range[] = { 0, 256 };
const float* ranges[] = { range };
Mat hist;
calcHist(&img, 1, 0, Mat(), hist, 1, histSize, ranges);
// 显示直方图
int hist_w = 512, hist_h = 400;
int bin_w = cvRound((double)hist_w / histSize[0]);
Mat hist_image(hist_h, hist_w, CV_8UC1, Scalar(0, 0, 0));
normalize(hist, hist, 0, hist_image.rows, NORM_MINMAX, -1, Mat());
for (int i = 1; i < histSize[0]; i++)
{
line(hist_image, Point(bin_w * (i - 1), hist_h - cvRound(hist.at<float>(i - 1))),
Point(bin_w * (i), hist_h - cvRound(hist.at<float>(i))), Scalar(255, 255, 255), 2, LINE_AA);
}
imshow("Histogram", hist_image);
waitKey(0);
return 0;
}
```
在这个示例中,我们首先使用 `imread()` 函数加载图像。然后,我们使用 `meanStdDev()` 函数计算图像的均值和标准差。接下来,我们使用 `calcHist()` 函数计算图像的直方图。最后,我们使用 `normalize()` 函数将直方图数据归一化并使用 `line()` 函数绘制直方图。
在实际使用中,您需要根据自己的需求进行相应的修改和调整。
阅读全文
相关推荐



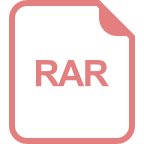

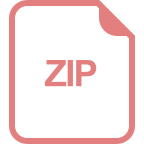



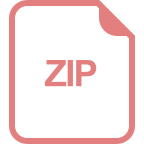
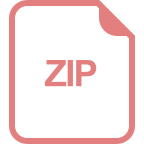
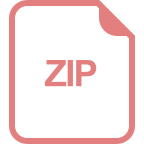
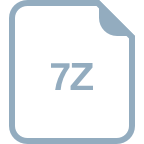