用c++写一个扫雷游戏
时间: 2023-12-25 09:01:01 浏览: 23
好的,下面是一个简单的扫雷游戏的 C++ 代码示例:
```c++
#include<iostream>
#include<cstdlib>
#include<ctime>
using namespace std;
const int ROW = 10; // 地图行数
const int COL = 10; // 地图列数
const int MINE_NUM = 10; // 地雷数量
int mine_map[ROW][COL] = {0}; // 地雷地图,0表示无地雷,1表示有地雷
int show_map[ROW][COL] = {0}; // 显示地图,0表示未翻开,-1表示地雷,1-8表示周围地雷数量
int remain = ROW * COL - MINE_NUM; // 剩余未翻开的格子数
// 随机生成地雷地图
void generate_mine_map(int row, int col, int mine_num)
{
int count = 0;
while (count < mine_num)
{
int x = rand() % row;
int y = rand() % col;
if (mine_map[x][y] == 0)
{
mine_map[x][y] = 1;
count++;
}
}
}
// 计算坐标(x,y)周围的地雷数量
int count_around_mines(int x, int y)
{
int count = 0;
for (int i = x - 1; i <= x + 1; i++)
{
for (int j = y - 1; j <= y + 1; j++)
{
if (i >= 0 && i < ROW && j >= 0 && j < COL && mine_map[i][j] == 1)
{
count++;
}
}
}
return count;
}
// 翻开坐标(x,y)周围的格子
void open_around(int x, int y)
{
for (int i = x - 1; i <= x + 1; i++)
{
for (int j = y - 1; j <= y + 1; j++)
{
if (i >= 0 && i < ROW && j >= 0 && j < COL && show_map[i][j] == 0)
{
show_map[i][j] = count_around_mines(i, j);
remain--;
if (show_map[i][j] == 0)
{
open_around(i, j);
}
}
}
}
}
// 显示地图
void show()
{
cout << " ";
for (int j = 0; j < COL; j++)
{
cout << j << " ";
}
cout << endl;
for (int i = 0; i < ROW; i++)
{
cout << i << " ";
for (int j = 0; j < COL; j++)
{
if (show_map[i][j] == 0)
{
cout << "* ";
}
else if (show_map[i][j] == -1)
{
cout << "X ";
}
else
{
cout << show_map[i][j] << " ";
}
}
cout << endl;
}
cout << "剩余未翻开的格子数:" << remain << endl;
}
int main()
{
srand(time(NULL)); // 初始化随机数种子
generate_mine_map(ROW, COL, MINE_NUM); // 随机生成地雷地图
show(); // 显示地图
int x, y;
while (remain > 0)
{
cout << "请输入翻开的坐标(x,y):";
cin >> x >> y;
if (mine_map[x][y] == 1)
{
cout << "很遗憾,你踩到地雷了!" << endl;
show_map[x][y] = -1;
show();
break;
}
else
{
show_map[x][y] = count_around_mines(x, y);
remain--;
if (show_map[x][y] == 0)
{
open_around(x, y);
}
show();
}
}
if (remain == 0)
{
cout << "恭喜你,扫雷成功!" << endl;
}
return 0;
}
```
这个示例中,首先通过 `generate_mine_map()` 函数随机生成地雷地图,然后在每一轮游戏中,通过 `show()` 函数显示当前的地图,等待玩家输入要翻开的坐标 `(x,y)`,如果该坐标上有地雷,则游戏失败;否则,通过 `count_around_mines()` 函数计算该坐标周围的地雷数量,并将其保存到 `show_map` 数组中,如果该坐标周围没有地雷,则通过 `open_around()` 函数递归地翻开周围的格子。最后,如果所有地雷都被找到,则游戏成功。
相关推荐
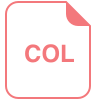










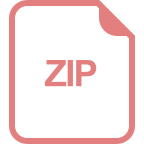