如何使用cJSON将string转化为json
时间: 2024-01-25 16:02:05 浏览: 183
对象?
你可以使用cJSON_Parse函数将字符串转换成JSON对象,例如:
```
#include <stdio.h>
#include "cJSON.h"
int main() {
const char *json_string = "{\"name\":\"John\", \"age\":30}";
cJSON *root = cJSON_Parse(json_string);
if (root != NULL) {
cJSON *name = cJSON_GetObjectItem(root, "name");
cJSON *age = cJSON_GetObjectItem(root, "age");
printf("Name: %s, Age: %d\n", name->valuestring, age->valueint);
}
cJSON_Delete(root);
return 0;
}
```
在这个例子中,我们将字符串`"{\"name\":\"John\", \"age\":30}"`转换成了JSON对象,并且通过`cJSON_GetObjectItem`函数获取了`"name"`和`"age"`字段的值。需要注意的是,使用完毕后需要调用`cJSON_Delete`函数释放内存。
相关问题
用C语言将txt文件转化为json文件
首先,你需要了解一些基本的C语言操作文件的知识,如何打开和关闭文件,读写文件等。
接下来,我们需要使用C语言中的JSON库来将txt文件转化为json文件。C语言中有很多JSON库可以使用,例如 cJSON,Jansson 等等。
下面是一个简单的示例代码,使用cJSON库将txt文件转化为json文件:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "cJSON.h"
int main()
{
FILE *fp_txt, *fp_json;
char txt_line[1024];
cJSON *json_root, *json_array, *json_item;
fp_txt = fopen("input.txt", "r");
fp_json = fopen("output.json", "w");
if (fp_txt == NULL || fp_json == NULL) {
printf("Error: cannot open file!\n");
return -1;
}
json_root = cJSON_CreateObject();
json_array = cJSON_CreateArray();
while (fgets(txt_line, sizeof(txt_line), fp_txt)) {
json_item = cJSON_CreateString(txt_line);
cJSON_AddItemToArray(json_array, json_item);
}
cJSON_AddItemToObject(json_root, "data", json_array);
char *json_str = cJSON_Print(json_root);
fprintf(fp_json, "%s", json_str);
free(json_str);
cJSON_Delete(json_root);
fclose(fp_txt);
fclose(fp_json);
return 0;
}
```
在这个代码中,我们使用了 cJSON 库来创建 JSON 对象,然后逐行读取 txt 文件的内容,将其添加到 JSON 数组中,并最终将整个 JSON 对象写入到 json 文件中。
注意,这只是一个简单的示例代码,实际应用中还需要处理更多的错误和异常情况。
CJSON 构建数组
CJSON是一个轻量级的JSON解析器和生成器,使用它可以方便地将JSON字符串转化为C语言中的数组、结构体或者指针类型,同时也可以将C语言数据转化为JSON字符串。下面是一个使用CJSON构建数组的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include "cJSON.h"
int main()
{
cJSON *root = cJSON_CreateArray(); // 创建一个空数组
// 往数组中添加三个元素,分别是字符串、整型和浮点数
cJSON_AddItemToArray(root, cJSON_CreateString("hello"));
cJSON_AddItemToArray(root, cJSON_CreateNumber(123));
cJSON_AddItemToArray(root, cJSON_CreateNumber(3.14));
char *json_str = cJSON_Print(root); // 将数组转换成JSON字符串
printf("%s\n", json_str);
cJSON_Delete(root); // 释放内存
free(json_str);
return 0;
}
```
运行上述代码,输出结果为:
```
["hello",123,3.140000]
```
阅读全文
相关推荐
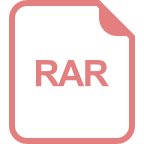
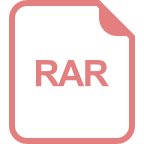
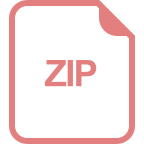
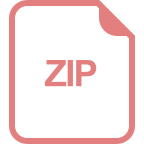
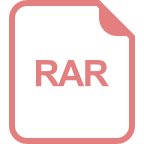
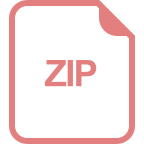
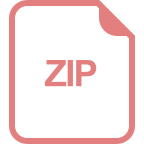
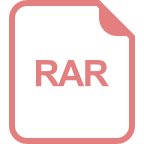
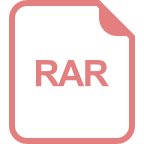
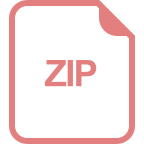
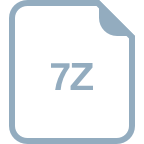
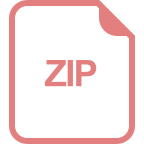
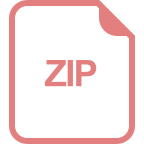
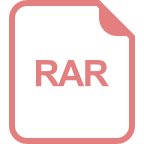
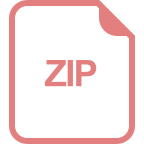
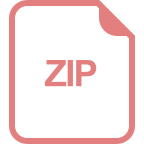