C语言使用cjson库,解析一个json文件,并将所有数据解析出来
时间: 2024-03-17 15:39:51 浏览: 71
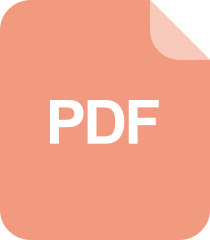
C语言cJSON库的使用教程,解析json数据格式.pdf
使用cjson库解析一个json文件并将所有数据解析出来可以分为以下几步:
1. 引入cjson库的头文件,如:`#include "cJSON.h"`
2. 读取json文件内容到一个字符串变量中,如:
```
char* json_content = NULL;
FILE* fp = fopen("example.json", "rb");
fseek(fp, 0, SEEK_END);
long file_size = ftell(fp);
rewind(fp);
json_content = (char*)malloc(file_size + 1);
fread(json_content, file_size, 1, fp);
fclose(fp);
json_content[file_size] = '\0';
```
3. 使用cJSON库的`cJSON_Parse()`函数解析json字符串,如:
```
cJSON* root = cJSON_Parse(json_content);
if (root == NULL) {
printf("Error before: [%s]\n", cJSON_GetErrorPtr());
}
```
4. 通过`cJSON_GetObjectItem()`函数获取json对象的成员变量,如:
```
cJSON* name = cJSON_GetObjectItem(root, "name");
const char* name_value = name->valuestring;
```
5. 遍历json对象的所有成员变量,如:
```
cJSON* item = NULL;
cJSON_ArrayForEach(item, root) {
const char* item_name = item->string;
if (cJSON_IsString(item)) {
const char* item_value = item->valuestring;
printf("%s = %s\n", item_name, item_value);
} else if (cJSON_IsNumber(item)) {
int item_value = item->valueint;
printf("%s = %d\n", item_name, item_value);
}
}
```
6. 释放cJSON对象的内存,如:
```
cJSON_Delete(root);
```
完整的代码示例可以参考以下代码:
```
#include "cJSON.h"
#include <stdio.h>
#include <stdlib.h>
int main() {
char* json_content = NULL;
FILE* fp = fopen("example.json", "rb");
fseek(fp, 0, SEEK_END);
long file_size = ftell(fp);
rewind(fp);
json_content = (char*)malloc(file_size + 1);
fread(json_content, file_size, 1, fp);
fclose(fp);
json_content[file_size] = '\0';
cJSON* root = cJSON_Parse(json_content);
if (root == NULL) {
printf("Error before: [%s]\n", cJSON_GetErrorPtr());
return 1;
}
cJSON* item = NULL;
cJSON_ArrayForEach(item, root) {
const char* item_name = item->string;
if (cJSON_IsString(item)) {
const char* item_value = item->valuestring;
printf("%s = %s\n", item_name, item_value);
} else if (cJSON_IsNumber(item)) {
int item_value = item->valueint;
printf("%s = %d\n", item_name, item_value);
}
}
cJSON_Delete(root);
free(json_content);
return 0;
}
```
阅读全文
相关推荐
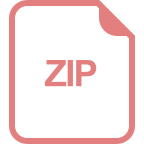
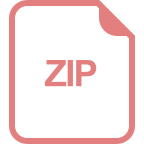















