c语言使用cjson库向json文件写入小数
时间: 2024-10-18 21:01:03 浏览: 42
CJSON是一个轻量级的JSON解析器和生成器,适用于C语言环境。如果你想用CJSON库将小数写入到JSON文件中,首先你需要包含cjson.h头文件,并链接相应的库。下面是一个简单的示例:
```c
#include "cJSON.h"
// 定义你要写的JSON数据结构
typedef struct {
char *name; // 对象键名
double value; // 小数值
} MyDecimal;
int main() {
cJSON *root = cJSON_CreateObject();
if (!root) {
printf("Error creating JSON object.\n");
return -1;
}
// 创建一个新的值节点,存储小数
MyDecimal decimal = {"quantity", 3.14};
cJSON *item = cJSON_CreateObject();
cJSON_AddStringToObject(item, "name", decimal.name);
cJSON_AddNumberToObject(item, "value", decimal.value);
// 把值节点添加到根对象
cJSON_AddItemToObject(root, "quantity", item);
// 将JSON写入文件
FILE* file = fopen("data.json", "w");
if (file == NULL) {
printf("Failed to open file for writing.\n");
cJSON_Delete(root); // 清理内存
return -1;
}
cJSON_Print(file, root); // 使用cJSON的打印功能
fclose(file);
cJSON_Delete(root); // 关闭并删除JSON对象
return 0;
}
```
在这个例子中,我们首先创建了一个JSON对象,然后添加了一个名为"quantity"的对象,其值为一个小数。最后我们将这个JSON字符串写入到"data.json"文件中。
阅读全文
相关推荐
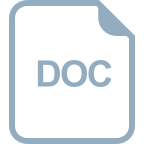
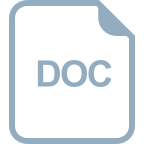
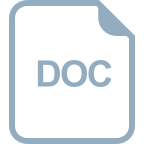







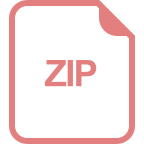
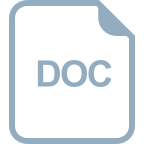
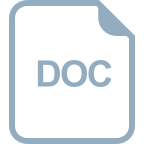
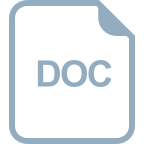



