c语言怎么通过cjson库向json文件写入小数
时间: 2024-10-09 19:16:38 浏览: 37
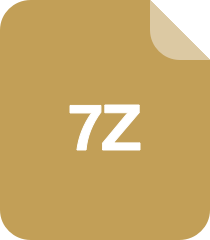
cJSON -轻量级的, 用于处理JSON数据的C语言库
在C语言中,你可以使用`cJSON`库将小数值写入JSON文件。首先,你需要包含`cJSON.h`头文件,并链接相应的库。下面是一个简单的示例,展示了如何创建一个JSON对象,添加一个键值对(包含一个小数),然后将其写入文件:
```c
#include <cJSON.h>
// 定义一个结构体来存储小数值
typedef struct {
char key[50];
double value;
} MyValue;
void write_to_json(const char* filename, const MyValue* data) {
cJSON *root = cJSON_CreateObject(); // 创建一个空的JSON对象
if (!root) {
printf("Error creating JSON object\n");
return;
}
// 添加小数值到JSON
cJSON* value = cJSON_CreateNumber(data->value); // 创建一个表示数字的节点
if (value == NULL) {
printf("Error creating number node\n");
cJSON_Delete(root);
return;
}
cJSON_AddItemToObject(root, "key", value); // 将数字节点添加到对象
// 将JSON字符串化并写入文件
FILE* file = fopen(filename, "w");
if (file == NULL) {
printf("Error opening file for writing\n");
cJSON_Delete(root);
return;
}
char* jsonString = cJSON_PrintUnformatted(root); // 将JSON转换为字符串
if (jsonString == NULL) {
printf("Error converting JSON to string\n");
fclose(file);
cJSON_Delete(root);
return;
}
fprintf(file, "%s\n", jsonString); // 写入文件
free(jsonString); // 释放字符串内存
fclose(file);
cJSON_Delete(root); // 删除不再需要的对象
}
int main() {
MyValue data = { "exampleKey", 3.14159 };
write_to_json("output.json", &data);
return 0;
}
```
在这个例子中,我们首先创建一个JSON对象,然后添加一个键值对("key": 3.14159)。注意,`cJSON_CreateNumber()`用于创建表示小数的节点。
阅读全文
相关推荐
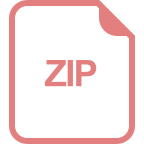





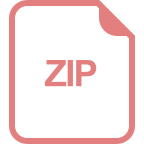
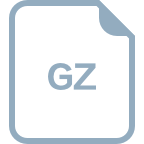
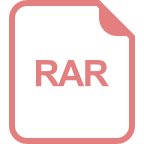
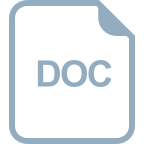
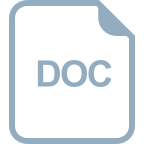
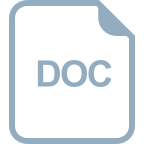
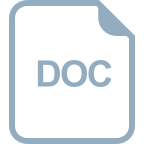



