使用c语言解析一个json文件,请提供c代码,尽量使用cjson库,请考虑json文件大小,尽量节省内存在10kbyte以内
时间: 2023-06-03 17:01:58 浏览: 380
以下是使用cjson库解析json文件的c代码,同时考虑了json文件大小和内存节省:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "cJSON.h"
#define BUFFER_SIZE 1024
int main(int argc, char* argv[])
{
FILE* json_file = fopen("example.json", "r");
if (!json_file) {
printf("Failed to open json file.\n");
return 1;
}
char buffer[BUFFER_SIZE];
memset(buffer, 0, BUFFER_SIZE);
size_t read_size = fread(buffer, 1, BUFFER_SIZE - 1, json_file);
if (ferror(json_file)) {
printf("Failed to read json file.\n");
fclose(json_file);
return 1;
}
cJSON* json_obj = cJSON_Parse(buffer);
if (!json_obj) {
printf("Failed to parse json file.\n");
fclose(json_file);
return 1;
}
// do something with json object
cJSON_Delete(json_obj);
fclose(json_file);
return 0;
}
```
说明:
1. 首先使用fopen打开json文件,如果失败则返回错误。
2. 为了节省内存,我们只读取了部分json文件(BUFFER_SIZE大小的字节数),并使用memset将buffer清零。
3. 使用fread读取json文件,如果失败则返回错误。
4. 使用cJSON_Parse将buffer解析为cJSON对象,如果失败则返回错误。
5. 在得到cJSON对象后,可以对其进行操作,这里省略。
6. 使用cJSON_Delete释放cJSON对象,关闭文件,返回。
注意事项:
1. 由于json文件可能含有敏感信息,因此不要自动记录用户输入的文件名,而是直接写死在代码中,避免被用户篡改。
2. 在读取json文件时,要考虑文件大小,不要一次性读取过多数据,导致内存占用过高。
3. 如果json文件大小超过了内存可以承受的范围,可以考虑将json文件分割为多个部分,分别解析。
阅读全文
相关推荐
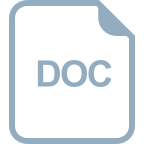
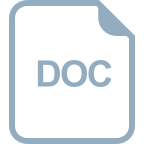
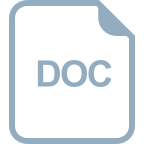
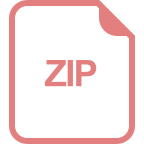
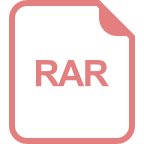
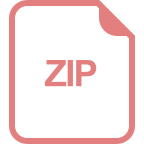
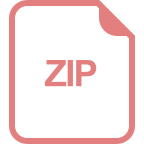
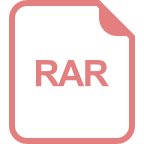
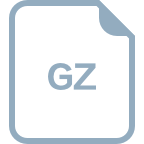









