c语言使用cjson库打开一个json文件并解析它
时间: 2024-12-23 09:24:15 浏览: 10
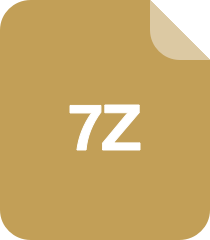
cJSON -轻量级的, 用于处理JSON数据的C语言库
要使用cJSON库在C语言中打开并解析JSON文件,首先确保已包含了cJSON库的头文件,并了解其基本用法。以下是一个简单的示例,展示了如何操作:
1. 包含必要的头文件[^1]:
```c
#include <cJSON.h>
```
2. 打开并读取文件[^2]:
```c
FILE* file = fopen("your_file.json", "r");
if (file == NULL) {
printf("Failed to open the file.\n");
return -1;
}
```
3. 分配内存以存储整个JSON字符串:
```c
size_t len = 0;
fseek(file, 0L, SEEK_END);
len = ftell(file);
rewind(file);
char* json_str = (char*)malloc(len + 1); // 加1是为了防止数组越界
fread(json_str, 1, len, file);
json_str[len] = '\0'; // 结束字符串
fclose(file);
```
4. 解析JSON字符串:
```c
cJSON* root = cJSON_Parse(json_str);
if (root == NULL) {
printf("Error parsing JSON string: %s\n", cJSON_GetErrorPtr());
free(json_str);
return -1;
}
```
5. 访问和处理解析后的JSON数据:
```c
// 示例:访问一个键值对
const char* key = "your_key";
const cJSON* value = cJSON_GetObjectItem(root, key);
if (value != NULL) {
printf("Value of '%s': %s\n", key, cJSON_Print(value));
} else {
printf("Key not found.\n");
}
// 清理内存
cJSON_Delete(root);
free(json_str);
```
请注意,你需要根据实际的JSON文件结构来访问和操作数据。
阅读全文
相关推荐
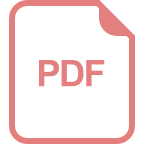
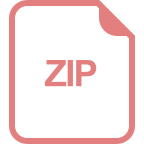





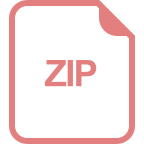
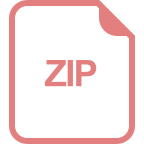
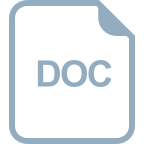
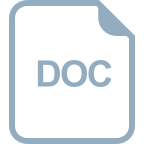
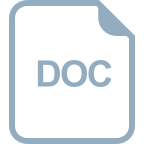
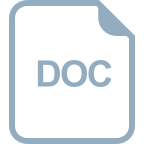



