c语言使用cjson库打开一个json文件并解析它示例
时间: 2024-12-23 08:24:35 浏览: 145
在C语言中使用cJSON库打开和解析JSON文件的步骤如下:
首先,确保包含了必要的头文件和初始化库:
```c
#include "cJSON.h"
#include <stdio.h>
```
1. **打开文件**:
```c
FILE* file = fopen("your_json_file.json", "r");
if (file == NULL) {
printf("Failed to open the JSON file.\n");
return;
}
```
2. **读取并解析JSON**:
创建一个临时的cJSON对象来存储读取的数据,然后逐行读取文件内容:
```c
cJSON *root = cJSON_Parse(file->buffer); // 假设已经设置了file的指针指向文件内容
if (root == NULL) {
printf("Error parsing JSON: %s\n", cJSON_GetErrorPtr());
fclose(file);
return;
}
```
3. **遍历和访问数据**:
你可以通过递归的方式访问JSON树的节点,如获取键值对:
```c
char *key = cJSON_GetObjectItem(root, "key_name")->valuestring; // 获取键名为"key_name"的值
```
4. **释放资源**:
在完成解析后,记得清理临时对象和关闭文件:
```c
cJSON_Delete(root); // 清理内存
fclose(file);
```
完整的示例代码:
```c
#include "cJSON.h"
#include <stdio.h>
// ... (上面提到的函数)
int main() {
FILE* file = fopen("your_json_file.json", "r");
if (!file) {
// 处理错误...
return -1;
}
cJSON *root = cJSON_Parse(file->buffer);
if (!root) {
fprintf(stderr, "Error parsing JSON: %s\n", cJSON_GetErrorPtr());
fclose(file);
return -1;
}
char *value = cJSON_GetObjectItem(root, "key_name")->valuestring;
// 使用value...
cJSON_Delete(root);
fclose(file);
return 0;
}
```
请注意,上述代码假设JSON文件已经被预加载到`file->buffer`中,实际应用中通常会分块读取文件而不是一次性加载整个文件。
阅读全文
相关推荐















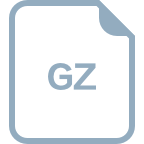
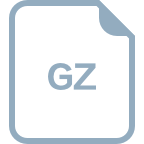

