c语言决胜21点(一)
时间: 2024-06-01 22:02:50 浏览: 14
好的,让我们开始设计一个用C语言实现的21点游戏吧!
首先,我们需要定义一些基本的规则:
1. 游戏玩家和电脑都会拿到两张牌,牌面点数加起来最接近21点的获胜;
2. 牌面点数从2到10的牌,点数就是牌面显示的数字;
3. J、Q、K的点数都为10点;
4. A牌可以算作1点或11点;
5. 游戏中不能超过21点,超过21点判定为输;
6. 玩家可以选择要牌或停牌,电脑也会自动发牌直到牌面点数大于等于17点。
有了基本规则后,我们就可以开始设计游戏流程了。
首先,我们需要定义一个结构体来表示一张牌:
```c
struct card {
char* face; // 牌面,如"2", "J", "Q", "K", "A"
int value; // 牌点数,2-10表示2-10点,J、Q、K为10点,A为1或11点
};
```
然后,我们需要定义一个数组来存储所有的牌:
```c
struct card deck[52] = {
{"2", 2}, {"3", 3}, {"4", 4}, {"5", 5}, {"6", 6}, {"7", 7},
{"8", 8}, {"9", 9}, {"10", 10}, {"J", 10}, {"Q", 10}, {"K", 10},
{"A", 11}, {"2", 2}, {"3", 3}, {"4", 4}, {"5", 5}, {"6", 6}, {"7", 7},
{"8", 8}, {"9", 9}, {"10", 10}, {"J", 10}, {"Q", 10}, {"K", 10},
{"A", 11}, {"2", 2}, {"3", 3}, {"4", 4}, {"5", 5}, {"6", 6}, {"7", 7},
{"8", 8}, {"9", 9}, {"10", 10}, {"J", 10}, {"Q", 10}, {"K", 10},
{"A", 11}, {"2", 2}, {"3", 3}, {"4", 4}, {"5", 5}, {"6", 6}, {"7", 7},
{"8", 8}, {"9", 9}, {"10", 10}, {"J", 10}, {"Q", 10}, {"K", 10},
{"A", 11}
};
```
接下来,我们需要定义一个函数来随机抽取一张牌:
```c
struct card draw_card() {
int rand_index = rand() % 52;
return deck[rand_index];
}
```
然后,我们需要定义一个函数来计算手中牌的点数:
```c
int calculate_points(struct card* hand, int num_cards) {
int total_points = 0;
int num_aces = 0;
for (int i = 0; i < num_cards; i++) {
total_points += hand[i].value;
if (strcmp(hand[i].face, "A") == 0) {
num_aces++;
}
}
while (num_aces > 0 && total_points > 21) {
total_points -= 10;
num_aces--;
}
return total_points;
}
```
接下来,我们需要定义一个函数来判断输赢:
```c
int determine_winner(int player_points, int dealer_points) {
if (player_points > 21) {
return -1; // 玩家输了
} else if (dealer_points > 21) {
return 1; // 玩家赢了
} else if (player_points > dealer_points) {
return 1; // 玩家赢了
} else if (player_points < dealer_points) {
return -1; // 玩家输了
} else {
return 0; // 平局
}
}
```
最后,我们需要定义一个主函数来控制游戏流程:
```c
int main() {
srand(time(NULL)); // 初始化随机数种子
struct card player_hand[10]; // 玩家手中的牌
struct card dealer_hand[10]; // 电脑手中的牌
int num_player_cards = 0; // 玩家手中牌的数量
int num_dealer_cards = 0; // 电脑手中牌的数量
int player_points = 0; // 玩家手中牌的点数
int dealer_points = 0; // 电脑手中牌的点数
// 发两张牌给玩家
player_hand[num_player_cards++] = draw_card();
player_hand[num_player_cards++] = draw_card();
// 发两张牌给电脑
dealer_hand[num_dealer_cards++] = draw_card();
dealer_hand[num_dealer_cards++] = draw_card();
// 显示玩家手中的牌和点数
printf("Your cards:\n");
for (int i = 0; i < num_player_cards; i++) {
printf("%s ", player_hand[i].face);
}
printf("\n");
player_points = calculate_points(player_hand, num_player_cards);
printf("Your points: %d\n", player_points);
// 玩家选择要牌或停牌
while (player_points < 21) {
char choice;
printf("Do you want to hit (h) or stand (s)? ");
scanf(" %c", &choice);
if (choice == 'h') {
player_hand[num_player_cards++] = draw_card();
printf("Your cards:\n");
for (int i = 0; i < num_player_cards; i++) {
printf("%s ", player_hand[i].face);
}
printf("\n");
player_points = calculate_points(player_hand, num_player_cards);
printf("Your points: %d\n", player_points);
} else {
break;
}
}
// 玩家输赢判断
if (player_points > 21) {
printf("Bust! You lose.\n");
return 0;
}
// 电脑自动发牌直到点数大于等于17点
while (dealer_points < 17) {
dealer_hand[num_dealer_cards++] = draw_card();
dealer_points = calculate_points(dealer_hand, num_dealer_cards);
}
// 显示电脑手中的牌和点数
printf("Dealer's cards:\n");
for (int i = 0; i < num_dealer_cards; i++) {
printf("%s ", dealer_hand[i].face);
}
printf("\n");
dealer_points = calculate_points(dealer_hand, num_dealer_cards);
printf("Dealer's points: %d\n", dealer_points);
// 判断输赢
int result = determine_winner(player_points, dealer_points);
if (result == 1) {
printf("You win!\n");
} else if (result == -1) {
printf("You lose!\n");
} else {
printf("It's a tie!\n");
}
return 0;
}
```
这就是一个简单的21点游戏的C语言实现,希望能对你有所帮助!
相关推荐
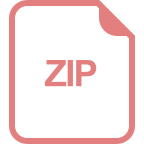
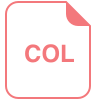














